How to Set Background Color for Flutter Column
In this blog post, we discuss something that many of us take for granted, setting the background color of a Column in Flutter. Though the Column widget doesn’t have a direct property for this, there are workarounds to help you achieve your desired look.
The Column Widget and Its Limitations
The Column widget in Flutter is perfect for aligning child widgets vertically. However, you’ll notice that the Column widget itself doesn’t have a color property. So, what can we do? Let’s explore.
Wrap with Container
The simplest approach to set a background color for your entire Column is to wrap it with a Container
widget and then use its color
property.
Container(
color: Colors.blue,
child: Column(
mainAxisSize: MainAxisSize.max,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Text('First child'),
Text('Second child'),
],
),
)
Here, the Container
acts as the background for the Column, and we’ve set its color to blue.
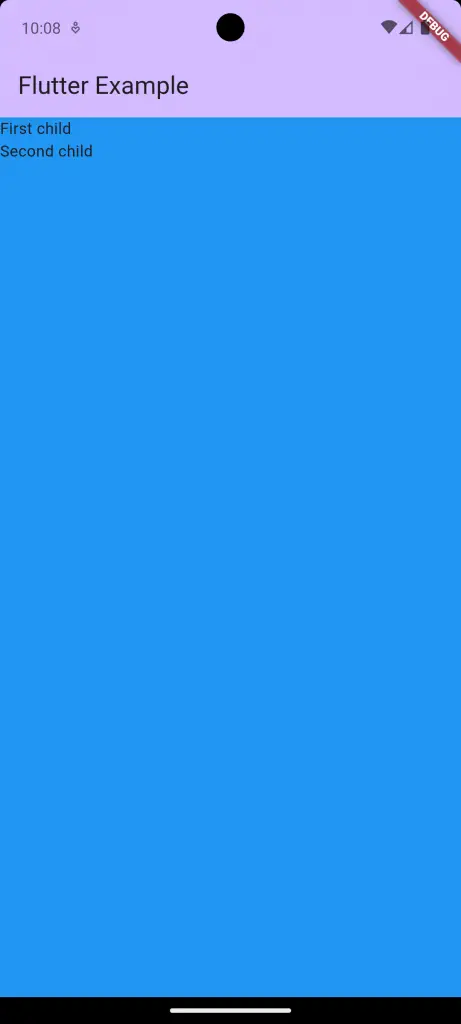
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
color: Colors.blue,
child: const Column(
mainAxisSize: MainAxisSize.max,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Text('First child'),
Text('Second child'),
],
),
),
);
}
}
Adding a background color to a Flutter Column might not be straightforward but with approaches like using a Container
, you can easily achieve the look you want.