How to Underline Text in Flutter
Sometimes, you may want to underline text to get the attention of the user. Let’s check how to add text with underlining in Flutter.
The TextStyle class is used for styling Text widgets in Flutter. Using the decoration property and TextDecoration class, you can underline your text easily.
const Text(
'Underlined Text',
style: TextStyle(
color: Colors.black
decoration: TextDecoration.underline,
),
)
If you want to underline only a specific word or sentence then you should make use of the RichText widget. See the following example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Underline text',
),
),
body: Center(
child: RichText(
text: const TextSpan(
text: 'Here is ',
style: TextStyle(fontSize: 30, color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'underlined',
style: TextStyle(
decoration: TextDecoration.underline,
)),
TextSpan(text: ' text!'),
],
),
)));
}
}
Following is the output.
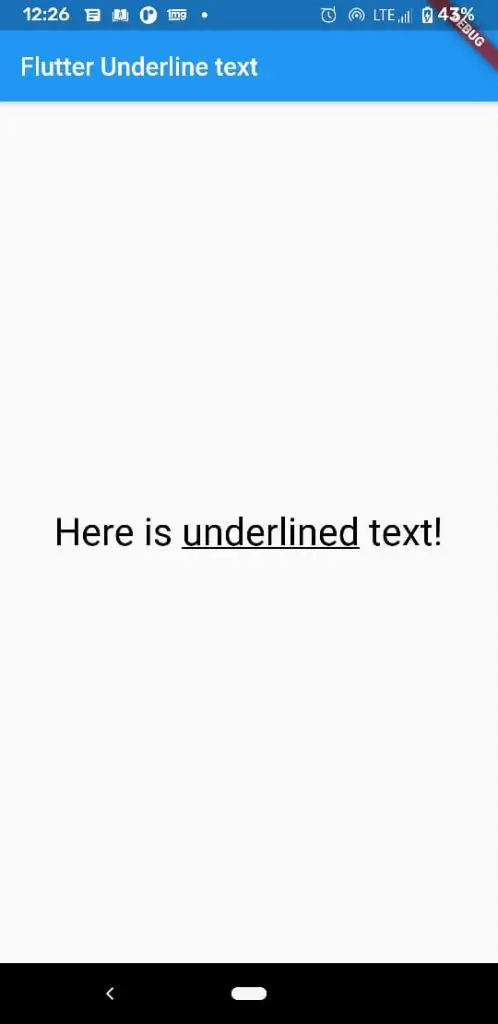
I hope you understand how to underline text in Flutter.