How to add Image Picker in React Native Expo
Expo is a set of tools and services built around React Native that makes it easier to develop and deploy mobile applications. In this blog post, let’s learn how to add an image picker using Expo.
I expect you have a react native project created using the Expo CLI. You can easily create an image picker using the expo-image-picker library.
Install the expo image picker using the following command.
npx expo install expo-image-picker
You can import the picker as given below.
import * as ImagePicker from 'expo-image-picker';
The ImagePicker.launchImageLibraryAsync method allows you to pick an image from the device. See the code snippet given below.
const pickImage = async () => {
let result = await ImagePicker.launchImageLibraryAsync({
mediaTypes: ImagePicker.MediaTypeOptions.Images,
allowsEditing: false,
});
console.log(result);
if (!result.canceled) {
setImage(result.assets[0].uri);
}
};
Following is the complete example in which you can pick an image when the button is pressed. And also, the selected image is also will be shown.
import React, { useState} from 'react';
import { Button, Image, View, StyleSheet } from 'react-native';
import * as ImagePicker from 'expo-image-picker';
export default function ImagePickerExample() {
const [image, setImage] = useState(null);
const pickImage = async () => {
let result = await ImagePicker.launchImageLibraryAsync({
mediaTypes: ImagePicker.MediaTypeOptions.Images,
allowsEditing: false,
});
console.log(result);
if (!result.canceled) {
setImage(result.assets[0].uri);
}
};
return (
<View style={styles.container}>
<Button title="Click Here" onPress={pickImage} />
{image && <Image source={{ uri: image }} style={styles.image} />}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems:'center',
justifyContent: 'center'
},
image: {
width: 250,
height: 250,
marginTop: 10
}
})
Following is the output.
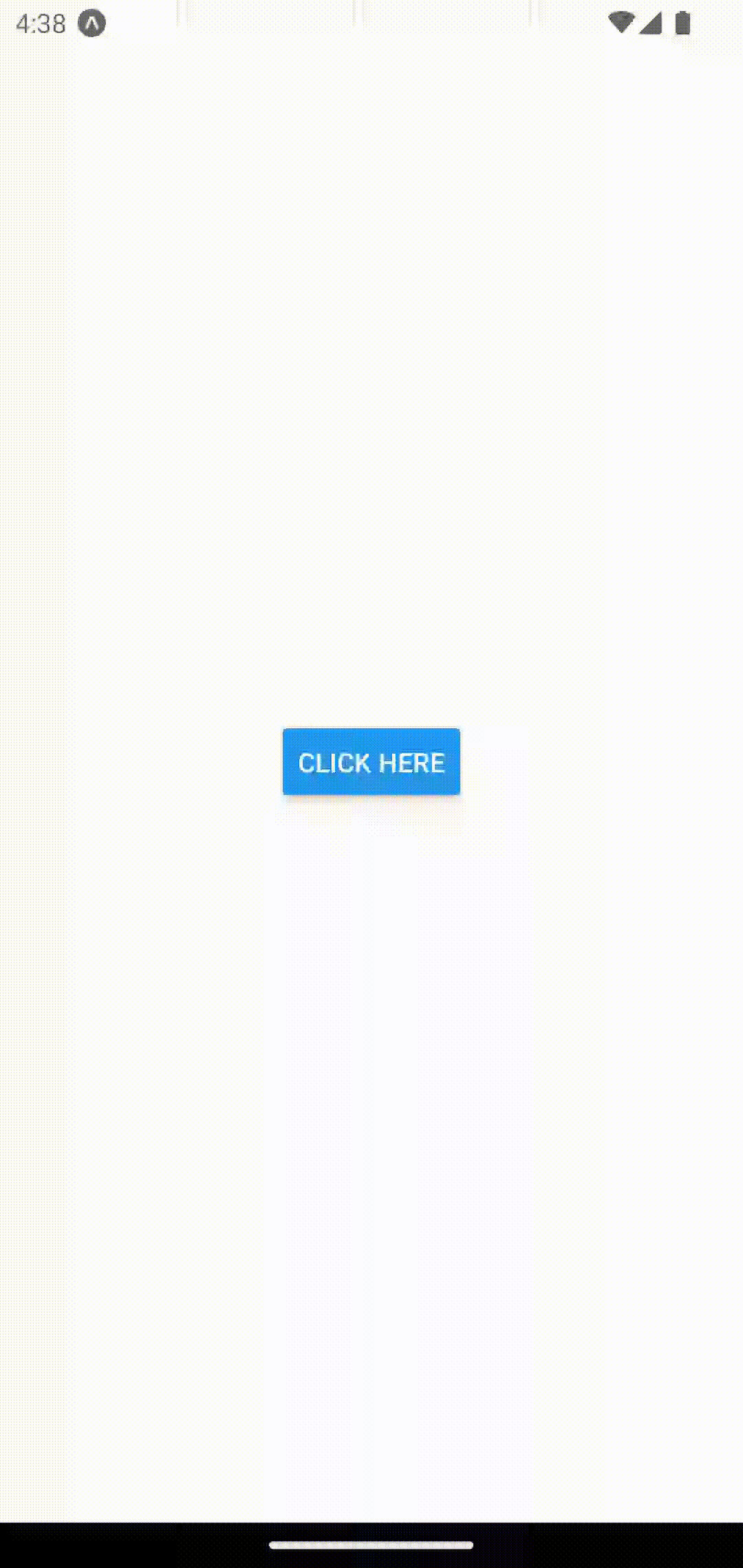
That’s how you add an image picker in react native expo.