How to Create Thumbnails for PDF Files in React Native
PDF is one of the most popular formats used for documents. As a result, you may have to add PDF support to your react native apps. In this blog post, let’s check how to create thumbnails for PDF files in react native.
We use a third party library named react native pdf thumbnail to generate thumbnail. You can install the library to your react native project using the following command.
npm install react-native-pdf-thumbnail
Following is the basic usage of the library.
import PdfThumbnail from "react-native-pdf-thumbnail";
// For iOS, the filePath can be a file URL.
// For Android, the filePath can be either a content URI, a file URI or an absolute path.
const filePath = 'file:///mnt/sdcard/myDocument.pdf';
const page = 0;
// The thumbnail image is stored in caches directory, file uri is returned.
// Image dimensions are also available to help you display it correctly.
const { uri, width, height } = await PdfThumbnail.generate(filePath, page);
// Generate thumbnails for all pages, returning an array of the object above.
const results = await PdfThumbnail.generateAllPages(filePath);
In the following react native example, we use one more library named react native document picker. It helps to pick PDF files from the phone. You can install it using the following command.
npm install react-native-document-picker
We pick a PDF file from the internal memory using react native document picker and show the thumbnail of the selected library using the react native pdf thumbnail library.
import React from 'react';
import {StyleSheet, Button, Text, Image, View} from 'react-native';
import DocumentPicker from 'react-native-document-picker';
import PdfThumbnail from 'react-native-pdf-thumbnail';
const App = () => {
const [thumbnail, setThumbnail] = React.useState('');
const [error, setError] = React.useState('');
const onPress = async () => {
try {
const {uri} = await DocumentPicker.pick({
type: [DocumentPicker.types.pdf],
});
const result = await PdfThumbnail.generate(uri, 0);
setThumbnail(result);
setError(undefined);
} catch (err) {
if (DocumentPicker.isCancel(err)) {
// User cancelled the picker, exit any dialogs or menus and move on
} else {
setThumbnail(undefined);
setError(err);
}
}
};
const thumbnailResult = thumbnail ? (
<>
<Image
source={thumbnail}
resizeMode="contain"
style={styles.thumbnailImage}
/>
<Text style={styles.thumbnailInfo}>uri: {thumbnail.uri}</Text>
<Text style={styles.thumbnailInfo}>width: {thumbnail.width}</Text>
<Text style={styles.thumbnailInfo}>height: {thumbnail.height}</Text>
</>
) : null;
const thumbnailError = error ? (
<>
<Text style={styles.thumbnailError}>Error code: {error.code}</Text>
<Text style={styles.thumbnailError}>Error message: {error.message}</Text>
</>
) : null;
return (
<View style={styles.container}>
<View style={styles.thumbnailPreview}>
{thumbnailResult}
{thumbnailError}
</View>
<Button onPress={onPress} title="Pick PDF File" />
</View>
);
};
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
padding: 20,
},
thumbnailPreview: {
padding: 20,
alignItems: 'center',
},
thumbnailImage: {
width: 200,
height: 200,
marginBottom: 20,
},
thumbnailInfo: {
color: 'darkblue',
},
thumbnailError: {
color: 'crimson',
},
});
Following is the output of the above example.
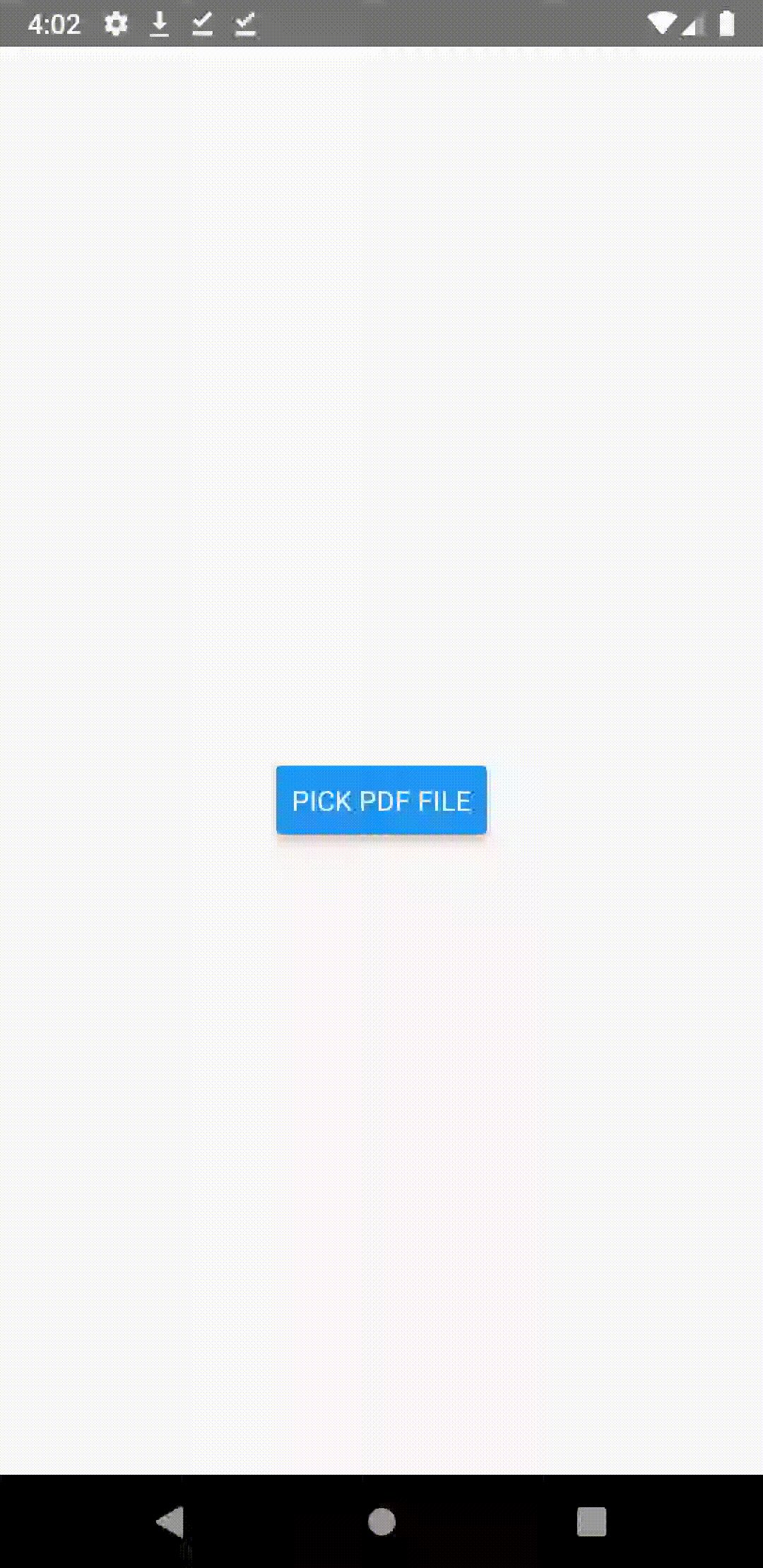