How to Create Icon Button with Text in React Native
Creating an aesthetically pleasing and functional icon button can make a huge difference in the user experience of your React Native app. In this react native tutorial, let’s learn how to add an icon button using react native vector icons.
When coming to icons, the react native vector icons library is a must have package for your react native app. It has a huge set of icons that can be used throughout your app.
Here, I am not covering the installation and setup of react native vector icons. I already have a tutorial on how to use react native vector icons and you can get all details there.
The react native vector icons library provides Icon.Button component and it is very useful when you need an icon button with text.
See the following code.
import {Text, View} from 'react-native';
import React from 'react';
import Icon from 'react-native-vector-icons/MaterialCommunityIcons';
function App() {
return (
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<Icon.Button
name="twitter"
backgroundColor="#4A99E9"
onPress={() => console.log('pressed')}>
<Text style={{color: 'white', fontSize: 15}}>Follow me</Text>
</Icon.Button>
</View>
);
}
export default App;
You will get the following output.
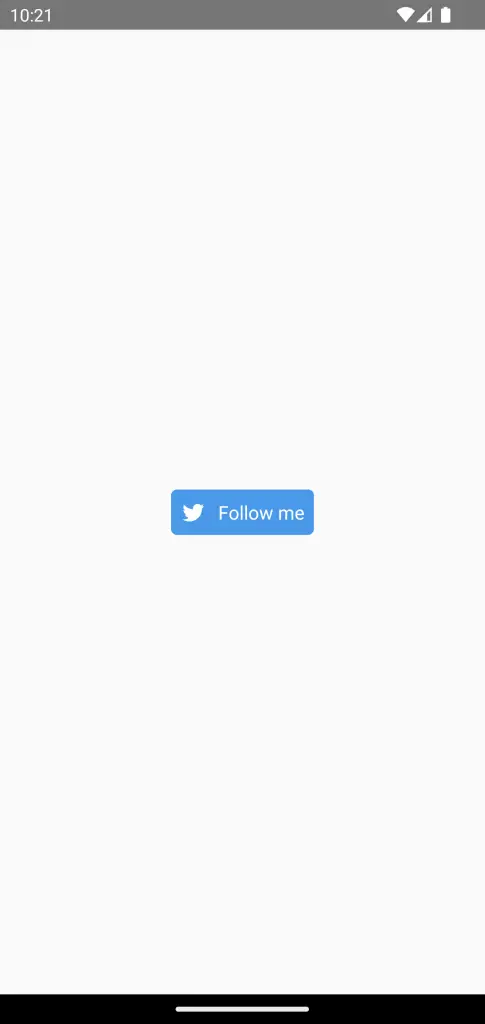
I hope this short react native tutorial on creating an icon button with text is helpful for you.