How to Change Text Size in iOS SwiftUI
In this blog post, we’re focusing on a fundamental aspect of app design – manipulating text size in SwiftUI. Understanding how to adjust the font size can make a significant difference in the aesthetics and user-friendliness of your application.
Basics: Change the Text Size
To alter the size of text in SwiftUI, we use the font()
modifier. SwiftUI provides a host of built-in font sizes from .largeTitle
to .caption
. Here’s a basic example:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, SwiftUI!")
.font(.title)
}
}
In this case, we’re displaying “Hello, SwiftUI!” in the size specified by .title
. SwiftUI dynamically adjusts the actual font size based on the device and user settings, providing a great out-of-the-box experience. There are many options to choose like .caption, .caption2, .footnote, .headline, .largeTitle, .subheadline, .title, .title2, .title3, etc.
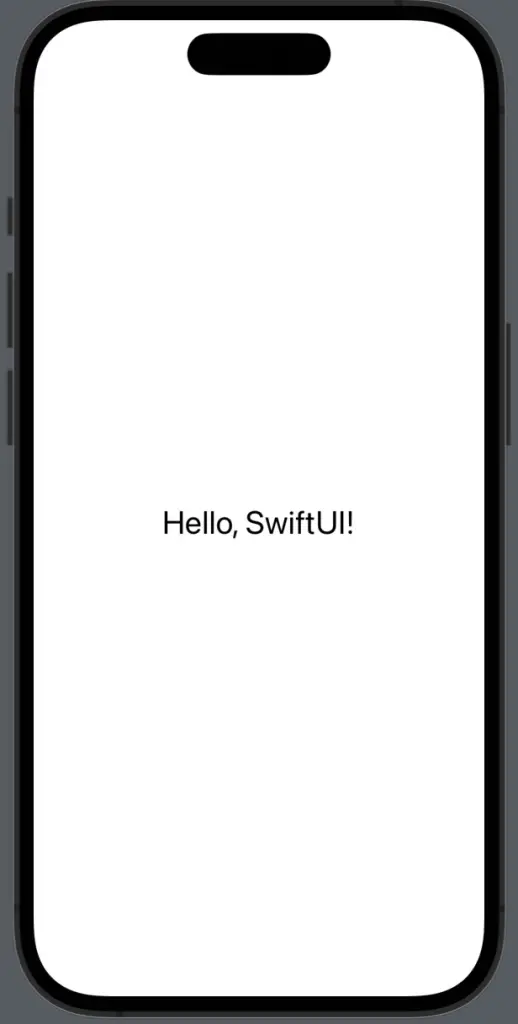
Custom Text Size
Apart from the predefined sizes, SwiftUI also allows us to specify a custom font size. This can be achieved using the .custom
style as follows:
Text("Hello, SwiftUI!")
.font(.system(size: 20))
In the example above, the text will appear with a custom font size of 20 points. The .system()
function here uses the system font with a specified size.
Dynamic Type Support
One great feature SwiftUI offers is support for Dynamic Type, allowing the text to adjust its size based on the user’s font size settings. This makes your app more accessible to a wider range of users.
Here’s how you enable dynamic type:
Text("Hello, SwiftUI!")
.font(.largeTitle)
.scaledToFit()
In this example, the .scaledToFit()
modifier ensures that the text scales according to the user’s system-wide font size settings.
Styled Text with Multiple Sizes
SwiftUI allows for the combination of different font sizes in a single Text view. Here’s how you can achieve it:
Text("Hello, ").font(.headline) +
Text("SwiftUI!").font(.title)
In the example above, “Hello, ” will display in .headline
size and “SwiftUI!” in .title
size, providing a subtle hierarchy to your text.
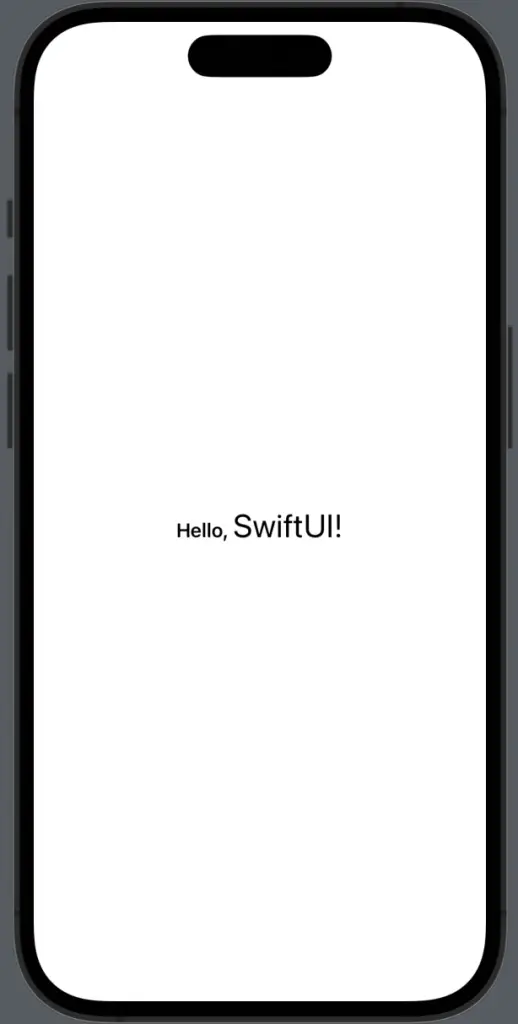
Whether you’re giving your app a style overhaul or you’re just tweaking to perfection, mastering the control of text size in SwiftUI is an essential step.
SwiftUI’s approach gives you a wealth of options from predefined styles to custom sizes, dynamic scaling, and even mixed styles in a single text view.
2 Comments