How to Disable FullScreenCover Animation in iOS SwiftUI
Animations are a core aspect of modern mobile app development, bringing fluidity and dynamism to user interfaces. SwiftUI offers a host of pre-built animations that get applied by default to certain UI elements, including the fullScreenCover
.
While this is beneficial most of the time, there are instances where you’d like to suppress these animations. In this blog post, let’s check how to disable animations when working with SwiftUI’s fullScreenCover
.
Default FullScreenCover Behavior
The Code
Let’s start by understanding the default behavior of fullScreenCover
using the following example:
import SwiftUI
struct ContentView: View {
@State private var isModalPresented = false
var body: some View {
Button("Show Full-Screen Modal") {
isModalPresented.toggle()
}
.fullScreenCover(isPresented: $isModalPresented) {
VStack{
Text("This is a full-screen modal")
Button("Hide Modal") {
isModalPresented.toggle()
}
}.frame(maxWidth: .infinity, maxHeight: .infinity).background(.yellow)
}
}
}
In this example, pressing the “Show Full-Screen Modal” button triggers a full-screen modal with an animation. The modal slides in from the bottom and slides out in the opposite direction when dismissed.
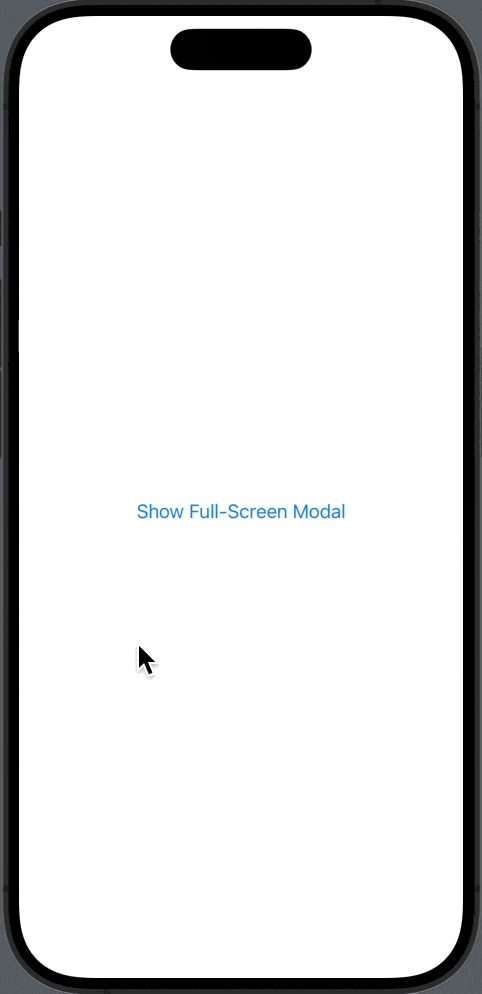
Disable FullScreenCover Animation
If you want to turn off these animations, SwiftUI offers a way to do that by manipulating transactions.
The Code
Here’s how to disable animations when showing and dismissing the fullScreenCover
:
import SwiftUI
struct ContentView: View {
@State private var isModalPresented = false
var body: some View {
Button("Show Full-Screen Modal") {
var transaction = Transaction()
transaction.disablesAnimations = true
withTransaction(transaction) {
isModalPresented.toggle()
}
}
.fullScreenCover(isPresented: $isModalPresented) {
VStack{
Text("This is a full-screen modal")
Button("Hide Modal") {
var transaction = Transaction()
transaction.disablesAnimations = true
withTransaction(transaction) {
isModalPresented.toggle()
}
}
}.frame(maxWidth: .infinity, maxHeight: .infinity).background(.yellow)
}
}
}
Code Explanation
var transaction = Transaction()
: A newTransaction
object is created.transaction.disablesAnimations = true
: This flag is set totrue
to disable animations for the transaction.withTransaction(transaction) { ... }
: The state-changing operation (isModalPresented.toggle()
) is wrapped insidewithTransaction
, which uses the settings specified intransaction
.
By manipulating the Transaction
, we have control over how state changes affect the UI, enabling us to disable animations when necessary.
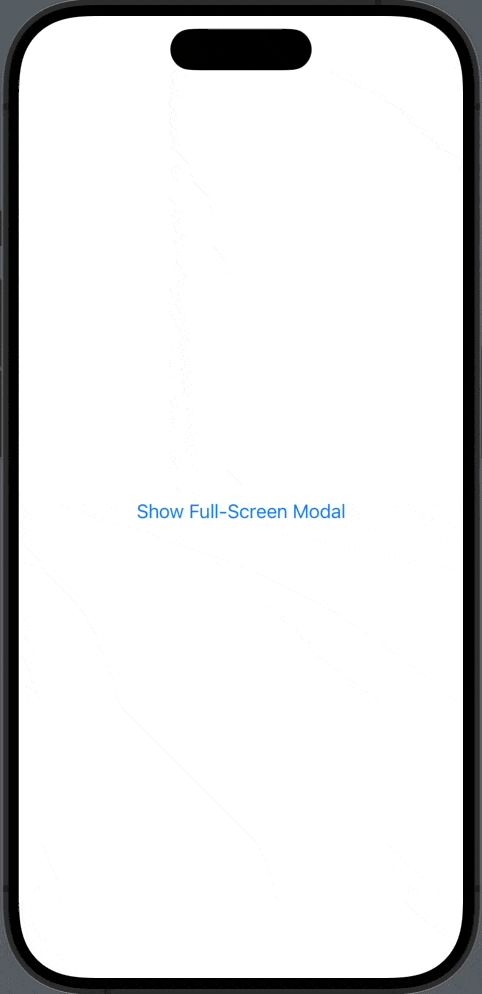
While animations usually improve the user experience, there might be cases where you’d like to suppress them. Whether it’s for performance reasons or simply because the UI feels cleaner without them, SwiftUI gives you the flexibility to disable animations using Transaction
.
One Comment