How to Automatically Dismiss a Sheet in iOS SwiftUI
In SwiftUI, sheets are commonly used to present secondary views or modals. While you can easily dismiss a sheet by tapping a button or dragging it down, there might be scenarios where you want the sheet to dismiss automatically after a certain period.
In this blog post, we’ll explore how to automatically dismiss a sheet in SwiftUI.
Default Behavior of Sheets
By default, a sheet in SwiftUI stays open until the user dismisses it manually or you change the state variable that controls its presentation.
Automatically Dismiss a Sheet
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Sheet") {
showModal = true
DispatchQueue.main.asyncAfter(deadline: .now() + 3) {
showModal = false
}
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a sheet!")
}.presentationDetents([.medium])
}
}
}
Code Explanation
DispatchQueue.main.asyncAfter(deadline: .now() + 3)
: We use Grand Central Dispatch (GCD) to schedule a closure that will run after a 3-second delay. Inside this closure, we setshowModal
tofalse
, which will dismiss the sheet..sheet(isPresented: $showModal)
: This is the standard way to present a sheet in SwiftUI. The sheet will show or hide based on the value of theshowModal
state variable.
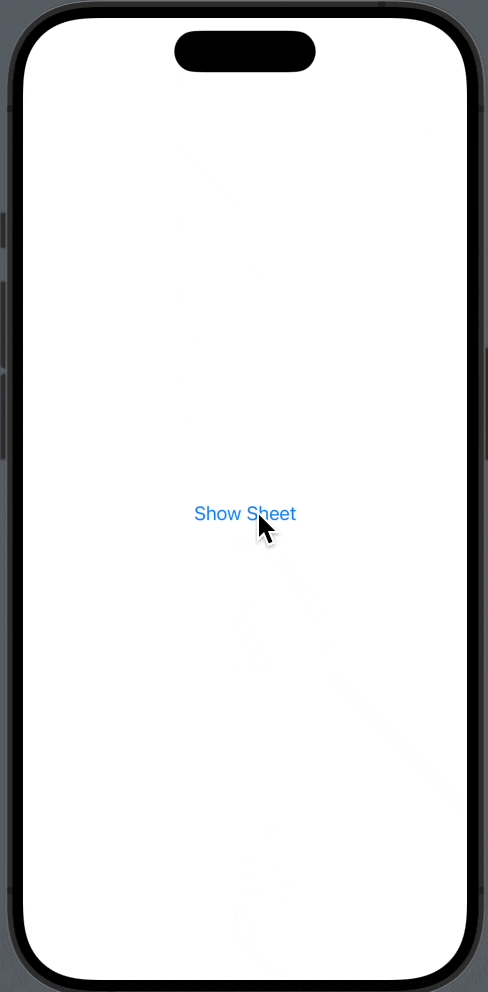
Use Cases for Automatic Dismissal
Here are some scenarios where automatically dismissing a sheet might be useful:
- Timed Alerts: If the sheet is being used to display a temporary alert or message.
- Loading Screens: If the sheet is showing a loading spinner and you want to dismiss it once the loading is complete.
- User Inactivity: If you want to dismiss the sheet after a certain period of user inactivity.
Automatically dismissing a sheet in SwiftUI is a straightforward task, thanks to the flexibility of the framework and the power of Grand Central Dispatch. Whether you’re displaying alerts, loading screens, or any other temporary information, automatic dismissal can be a useful feature to implement.