How to Change ProgressView Background Color in iOS SwiftUI
Progress indicators are essential UI elements that provide feedback on ongoing tasks. While SwiftUI’s ProgressView
offers various customization options, changing the background color isn’t straightforward.
In this blog post, we’ll explore different methods to set the background color of a ProgressView
in SwiftUI.
What is a ProgressView?
A ProgressView
in SwiftUI is a UI element that displays the progress of an ongoing task. It can be either determinate, showing a specific percentage of completion, or indeterminate, displaying a general waiting indicator.
Use Background Modifier
Example: Basic Background Color
The simplest way to set a background color is by using the .background
modifier.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView().padding()
.background(Color.yellow)
}
}
The .background
modifier sets the background color of the ProgressView
to red.
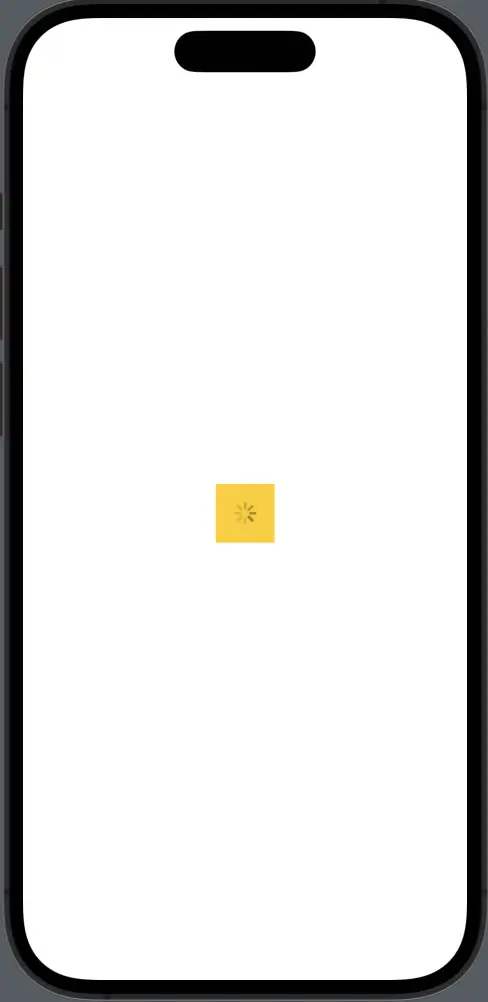
Use Overlay for Circular ProgressView
Example: Circular Background
For a circular ProgressView
, you can use the .overlay
modifier to add a background.
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView().padding()
.overlay(
Circle().fill(Color.yellow).opacity(0.5)
)
}
}
The .overlay
modifier adds a green circle behind the white circular ProgressView
. This way, the background color is set only for the progress indicator, not the entire view.
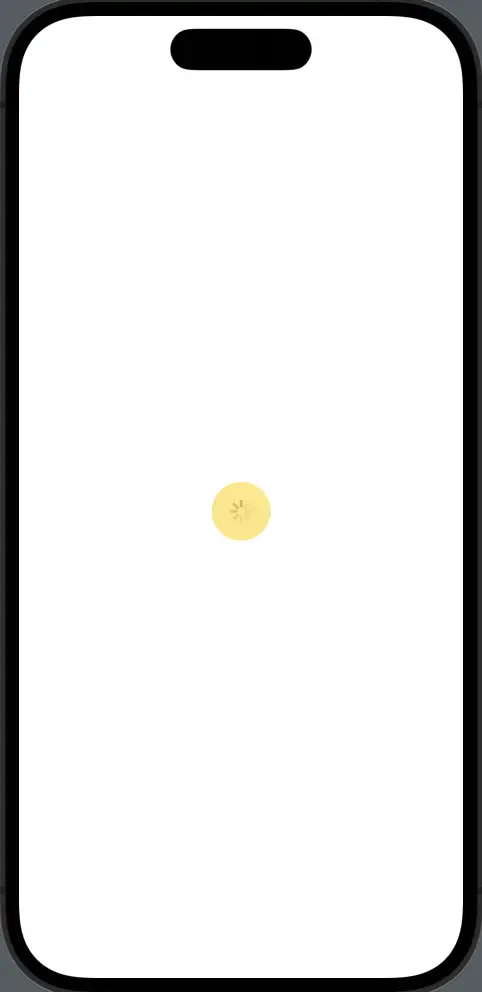
Considerations
- Visibility: Make sure the background color and the progress indicator color have enough contrast for better visibility.
- User Experience: Always consider the overall layout and design when changing the background color to ensure it fits well and provides a good user experience.
While SwiftUI doesn’t provide a built-in way to change the background color of ProgressView
, you can achieve this using various methods like .background
and .overlay
. Each method has its own advantages and use-cases, offering you the flexibility to choose the best fit for your app.