How to Use ProgressView as Overlay in iOS SwiftUI
In many scenarios, you may want to display a progress indicator as an overlay on top of another view, such as an image or a button. This is particularly useful for indicating the loading status of dynamic content.
In this blog post, we’ll explore how to create a ProgressView
overlay in SwiftUI, complete with examples and best practices.
What is a ProgressView?
A ProgressView
in SwiftUI is a UI element that displays the progress of an ongoing task. It can be either determinate, showing the exact percentage of completion, or indeterminate, displaying a general waiting indicator.
Basic ProgressView Overlay
Example: Overlay on an Image
Here’s a simple example of a ProgressView
overlay on an image:
import SwiftUI
struct ContentView: View {
var body: some View {
Image("clouds")
.overlay(
ProgressView().controlSize(.large).tint(.red)
)
}
}
The ProgressView
is added as an overlay on top of an Image
view. The controlSize
and tint
modifiers are used to style the progress indicator.
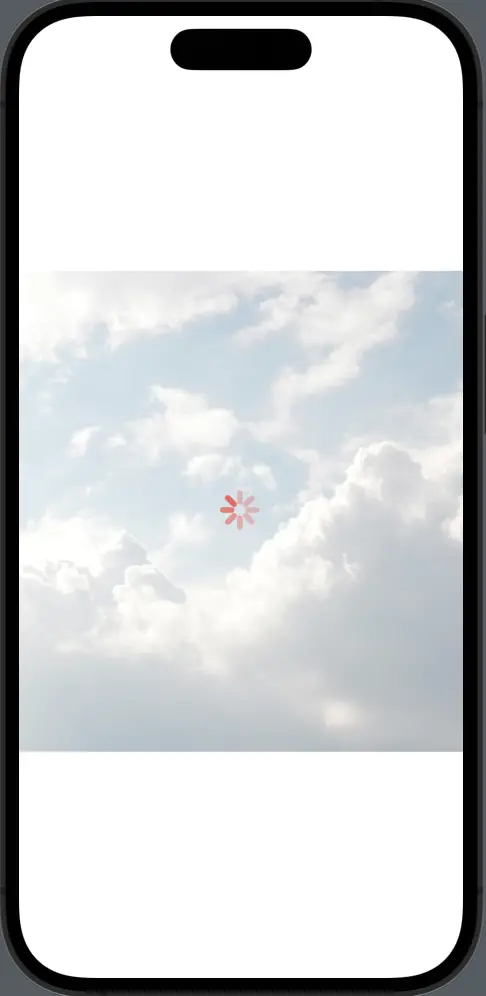
Conditional Overlays
Example: Show/Hide ProgressView Overlay
You can conditionally display the ProgressView
overlay based on a state variable:
@State private var isLoading: Bool = true
Image("example_image")
.overlay(
Group {
if isLoading {
ProgressView()
}
}
)
The ProgressView
overlay will only be displayed when the isLoading
state variable is set to true
.
Advanced Usage
ProgressView Overlay with Label
You can also add a label to the ProgressView
overlay for additional context:
import SwiftUI
struct ContentView: View {
var body: some View {
Image("clouds")
.overlay(
ProgressView("Loading...").controlSize(.large).tint(.red)
)
}
}
The label “Loading…” provides additional context to the user, indicating that the content is currently loading.
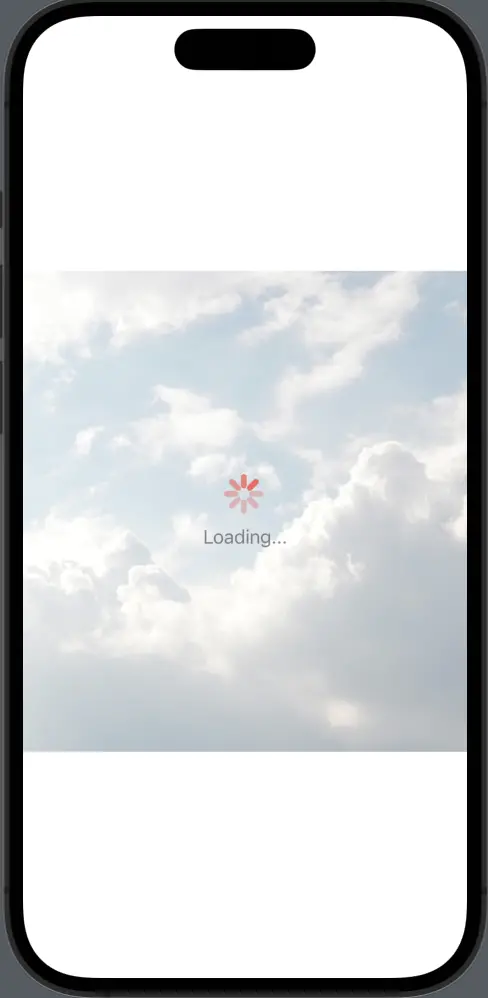
Using ProgressView
as an overlay in SwiftUI is a powerful way to indicate the loading or progress status of a task directly on the content. Whether you’re adding a simple overlay, customizing its appearance, or conditionally displaying it based on a state variable, SwiftUI offers the flexibility to meet your needs.