How to Create Shape Masks in SwiftUI
In the world of SwiftUI, a mask is a way to control the visibility of views. It’s an incredibly powerful tool that can be used to create custom shapes, animate changes, or simply to add an aesthetic touch to your UI.
In this blog post, we’ll focus on how to use shape masks to create visually stunning interfaces in SwiftUI.
What is a Shape Mask?
A mask in SwiftUI is a view that determines the transparency of another view. Views that are under opaque parts of the mask are visible, while views under transparent parts are invisible. This technique allows you to clip the view to the shape of the mask.
Create a Basic Shape Mask
Let’s start with the basics. Here’s how you can apply a simple circle mask to an image.
Image("dog")
.resizable()
.mask(Circle())
In this example, the mask()
modifier clips the Image
view to a Circle
shape. The resulting view will display the image only where the circle is opaque.
Use Masks for Creative Designs
Shape masks can be used not just for images, but for any view, to create intricate designs.
import SwiftUI
struct ContentView: View {
var body: some View {
Rectangle()
.fill(LinearGradient(gradient: Gradient(colors: [.blue, .green]), startPoint: .top, endPoint: .bottom))
.frame(width: 300, height: 200)
.mask(Text("SwiftUI")
.font(Font.system(size: 72).weight(.bold)))
}
}
In the above code, we create a Rectangle
with a linear gradient fill. Then, we apply a mask using a bold “SwiftUI” Text
view. The text acts as a mask, and the gradient is visible only where the text is placed.
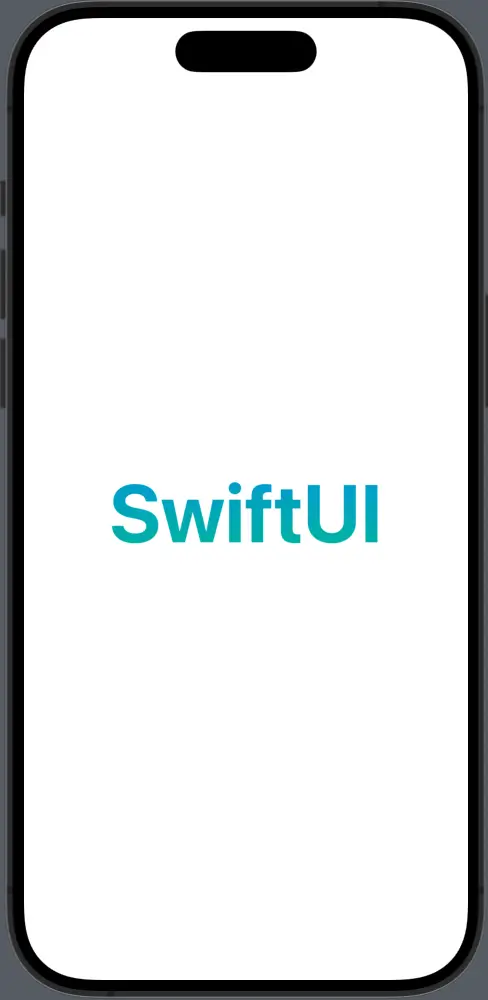
Custom Shapes as Masks
SwiftUI allows you to define custom shapes that can be used as masks. This is particularly useful when you want a non-standard shape to define the visible area of a view.
struct Triangle: Shape {
func path(in rect: CGRect) -> Path {
var path = Path()
path.move(to: CGPoint(x: rect.midX, y: rect.minY))
path.addLine(to: CGPoint(x: rect.minX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY))
path.closeSubpath()
return path
}
}
Image("avatar")
.resizable()
.frame(width: 200, height: 200)
.mask(Triangle())
The custom Triangle
shape is used as a mask for an image, resulting in the image being displayed only in the triangular area.
Shape masks in SwiftUI are a fantastic way to add depth, complexity, and visual interest to your app’s UI. By using masks, you can clip views to specific shapes, create custom designs, and even animate changes for dynamic effects.
Whether you’re masking images, text, or gradients, the power of shape masks can elevate your UI design to the next level.