How to Change TextField Border Color in iOS SwiftUI
The appearance of text fields is a significant part of the user interface in any application. SwiftUI provides simple ways to customize various UI components, including the TextField. Specifically, we’ll look at how to set a custom border color for a TextField using the border
modifier.
How to Customize TextField Border with border
Modifier
Below is an example that demonstrates how to create a TextField with a customized border color using the border
modifier:
struct ContentView: View {
@State private var text = ""
var body: some View {
TextField("Enter text", text: $text)
.padding(10)
.border(Color.blue, width: 2)
.padding()
}
}
Key Components of the Code
1. TextField
The TextField is set up with a placeholder “Enter text” and is bound to the text
state variable, which stores the user’s input.
2. Padding
Padding is applied to provide some space around the TextField, giving it a more pleasant appearance within the layout.
3. Border
The border
modifier is applied to the TextField, setting the border color to blue and the border width to 2.
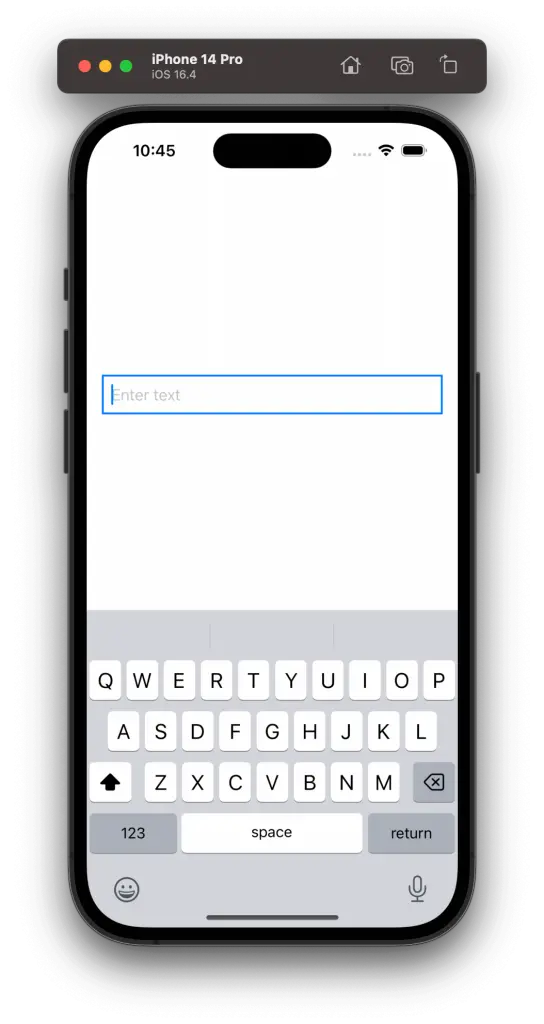
The border
modifier in SwiftUI provides a straightforward way to customize the border color and width of a TextField. By combining this with other modifiers like padding
you can create a unique and visually appealing text input component that fits your app’s design.