SwiftUI: LazyVGrid vs LazyHGrid
In the SwiftUI world, understanding the difference between LazyVGrid and LazyHGrid is important. Both areuseful tools for creating dynamic and flexible layouts. This post aims to help you understand the key differences between these two grid types and when to use them.
What is LazyVGrid?
LazyVGrid is a SwiftUI view that arranges its child views in a grid that scrolls vertically. The grid creates items as they become visible, hence optimizing performance, particularly when dealing with large sets of data.
LazyVGrid Example
Here’s a simple example of a LazyVGrid which displays a list of numbers in two columns:
struct ContentView: View {
let gridItems = [GridItem(.flexible()), GridItem(.flexible())]
var body: some View {
ScrollView {
LazyVGrid(columns: gridItems, spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 100, height: 100)
.background(Color.orange)
.cornerRadius(10)
}
}
}
}
}
In this example, the numbers 1 through 50 are displayed in an orange box. The grid will scroll vertically.
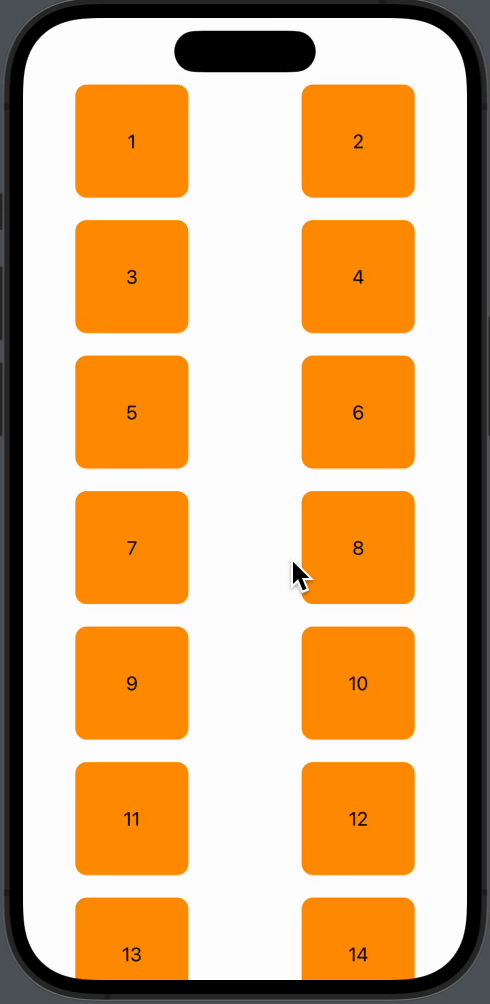
What is LazyHGrid?
In contrast, LazyHGrid is a SwiftUI view that arranges its child views in a grid that scrolls horizontally. Similar to LazyVGrid, it also only creates items as they become visible, offering the same performance optimization.
LazyHGrid Example
Now, let’s look at an example of a LazyHGrid which displays a list of numbers in two rows:
struct ContentView: View {
let gridItems = [GridItem(.flexible()), GridItem(.flexible())]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: gridItems, spacing: 20) {
ForEach(1...50, id: \.self) { index in
Text("\(index)")
.frame(width: 100, height: 100)
.background(Color.blue)
.cornerRadius(10)
}
}
}
}
}
In this example, the numbers 1 through 50 are displayed in a blue box. The grid will scroll horizontally.
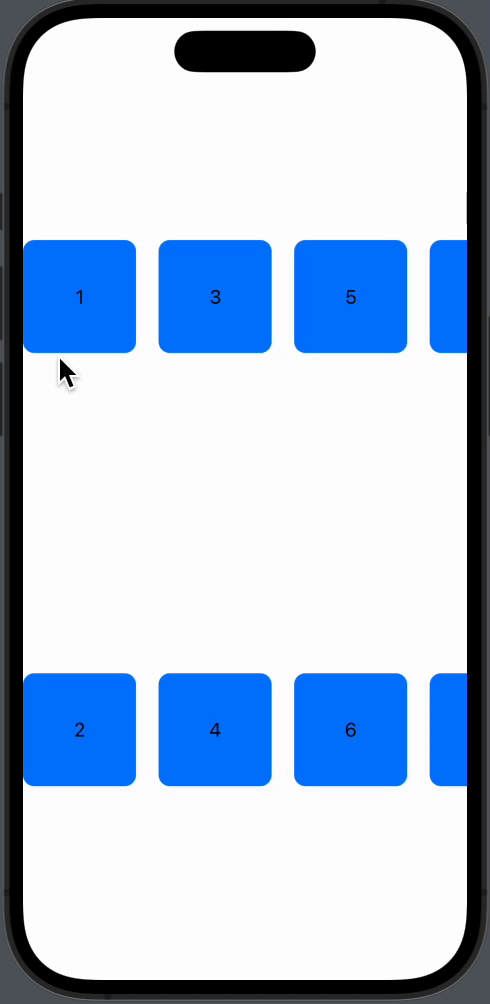
Differences Between LazyVGrid and LazyHGrid
Despite their similarities, the main difference between LazyVGrid and LazyHGrid lies in the scrolling direction and layout. LazyVGrid allows vertical scrolling with multiple columns, while LazyHGrid enables horizontal scrolling with multiple rows.
This means that if you want to display a collection of items in columns that the user can scroll through vertically, you should use LazyVGrid. On the other hand, if you want your user to scroll horizontally through rows of items, you should use LazyHGrid.
When to Use LazyVGrid or LazyHGrid?
Choosing between LazyVGrid and LazyHGrid ultimately depends on the layout needs of your application.
If you want to display data such as a photo gallery where users can scroll vertically through multiple columns of images, then LazyVGrid is a good choice. It’s also ideal for presenting items in a grid-like pattern that scrolls up and down.
On the other hand, if you want to display data like a list of books where users can scroll horizontally to see more items, then LazyHGrid is the better choice. It’s ideal for creating carousel-like layouts with rows of data that users can scroll from side to side.
In summary, both LazyVGrid and LazyHGrid are powerful tools for SwiftUI developers. Understanding their differences and when to use each can help you create more dynamic, flexible, and efficient layouts. Assess the needs of your application, and choose the grid that best fits those requirements.