How to Add an Icon to iOS SwiftUI TextField
Including icons in text fields can enhance the user interface by providing visual cues about the content or purpose of the field. For example, a search icon can denote a search field, and an email icon can mark an email input field.
This blog post will cover how to add an icon to a SwiftUI TextField, making the text input area more interactive and visually appealing.
Add an Icon to a SwiftUI TextField
Example: Email TextField with Icon
Here is an example demonstrating how to add an email icon to a TextField in SwiftUI:
struct ContentView: View {
@State private var email = ""
var body: some View {
HStack {
Image(systemName: "envelope")
.foregroundColor(.gray)
TextField("Enter email", text: $email)
}
.padding()
}
}
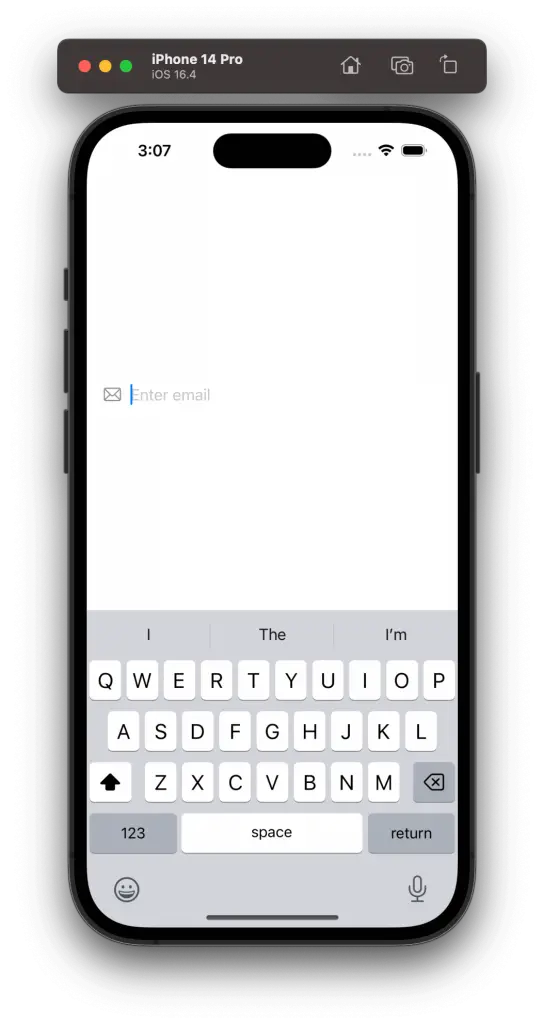
Explanation
Let’s break down the above code to understand how the icon is added to the TextField:
@State
Property Wrapper
The @State
property is used to bind the TextField to a variable, allowing it to store and react to changes in the text input.
HStack
An HStack
is used to place the icon and the TextField horizontally next to each other.
Image
An Image
view with the system name "envelope"
is used to display the email icon. You can customize the icon by changing the system name or using a custom image.
TextField
The TextField is created with a placeholder "Enter email"
and is bound to the email
state variable.
Padding
Padding is added around the HStack
to provide space and give a pleasant appearance.
Customize the TextField
You can further customize the TextField by adding a border using overlay.
struct ContentView: View {
@State private var email = ""
var body: some View {
HStack {
Image(systemName: "envelope")
.foregroundColor(.gray)
TextField("Enter email", text: $email)
}
.padding(20)
.overlay(
RoundedRectangle(cornerRadius: 6)
.stroke(Color.gray, lineWidth: 1)
.padding()
)
}
}
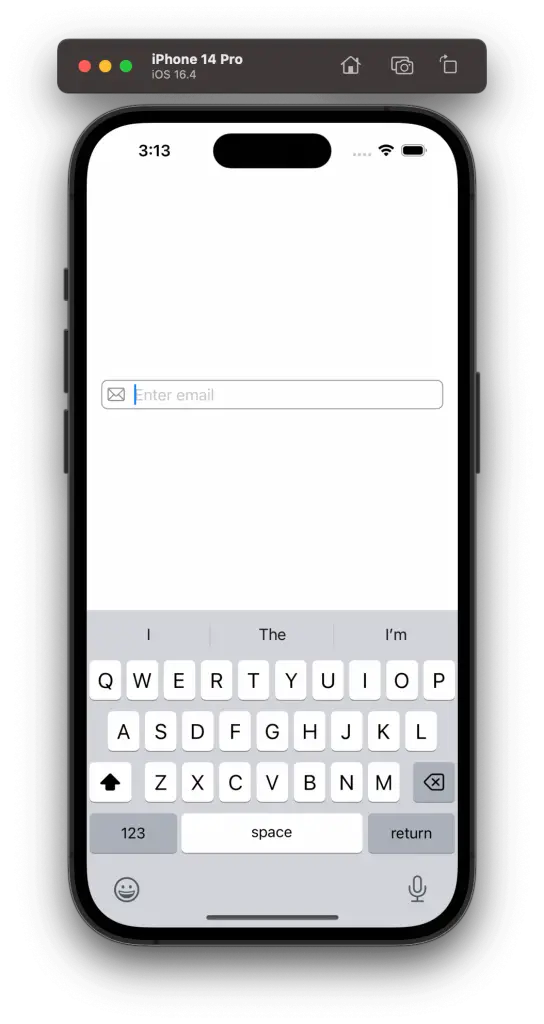
Adding icons to SwiftUI TextFields helps create a visually appealing and intuitive interface. By placing an Image
view alongside a TextField inside an HStack
, you can easily add icons corresponding to the text field’s purpose.
Customizing the icon’s appearance ensures that it aligns well with your app’s overall design.