How to Add a Clear Button to iOS SwiftUI TextField
User experience can be significantly enhanced by the addition of small, intuitive features, such as a clear button inside a text field. SwiftUI does not provide an in-built solution for this, but with some creative implementation, you can achieve it.
This blog post will guide you through adding a clear button to a SwiftUI TextField, complete with a rounded border style.
Create a Clear Button for TextField
Below is an example code snippet that demonstrates how to create a clear button inside a SwiftUI TextField:
struct ContentView: View {
@State private var text = ""
var body: some View {
HStack {
TextField("Enter text", text: $text)
.padding()
.textFieldStyle(.roundedBorder)
.overlay(
Button(action: {
text = ""
}) {
Image(systemName: "xmark.circle.fill")
.opacity(text.isEmpty ? 0 : 1).padding()
}
.padding(),
alignment: .trailing
)
}
}
}
Code Explanation
@State
Property
The @State
property wrapper is used to hold the text entered by the user. It enables the view to re-render as the text changes.
TextField with Rounded Border
The TextField is created with a placeholder and binding to the text
state variable. The .textFieldStyle(.roundedBorder)
modifier adds a rounded border to the TextField.
Overlay
The overlay
modifier is used to overlay the clear button on the TextField, positioning it at the trailing edge.
Button
A Button with an action that clears the text field by setting text
to an empty string.
Image with Opacity
An “X” symbol represented by Image(systemName: "xmark.circle.fill")
is used as the clear button’s appearance, with its opacity set to 0 if the text is empty.
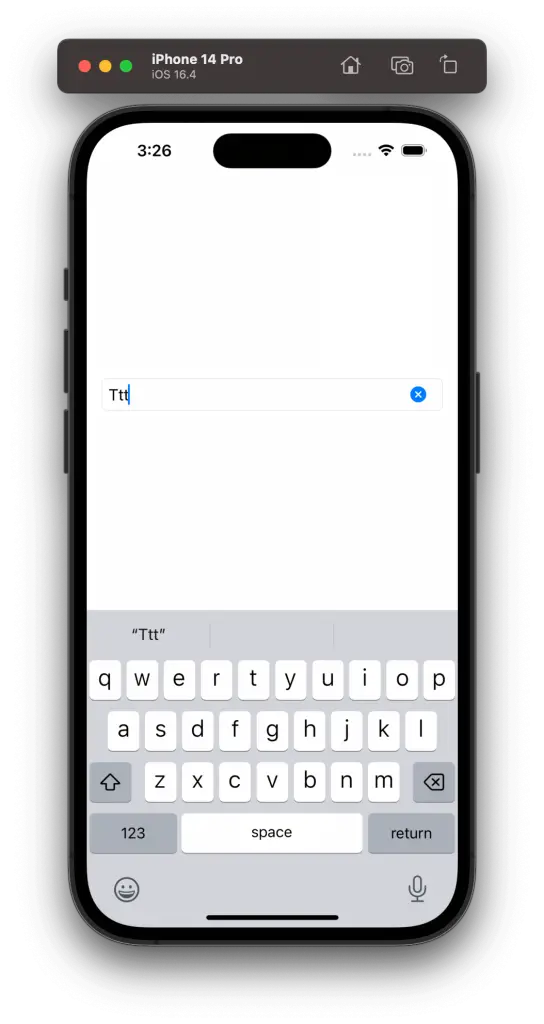
Styling and Customization
You can further style the clear button by modifying the Image, such as changing its color or size. Additional padding is added to both the image and the button for proper alignment and appearance.
The addition of a clear button in a text input field is an intuitive way to enhance the user experience. Using SwiftUI’s modifiers like overlay
and textFieldStyle
, you can create this feature in your app.
The provided code snippet guides you through creating a clear button inside a TextField with a rounded border style, adding a polished touch to your application’s text input fields.