How to Add Form Title in iOS SwiftUI
Titles play a significant role in enhancing user experience by providing context and organization to your Forms in SwiftUI. Whether it’s section titles or a navigation bar title, they make your form more readable and user-friendly.
This post will explore how to add titles to SwiftUI Forms, focusing on section titles and navigation titles.
Add Section Titles
Section titles help categorize different parts of the form, making it more intuitive for users. Here’s an example:
import SwiftUI
struct ContentView: View {
@State private var username = ""
@State private var email = ""
var body: some View {
Form {
Section(header: Text("User Information")) {
TextField("Username", text: $username)
TextField("Email", text: $email)
}
Section(header: Text("Preferences")) {
Toggle("Enable Notifications", isOn: .constant(true))
Picker("Theme", selection: .constant(1)) {
Text("Light").tag(1)
Text("Dark").tag(2)
}
}
}
}
}
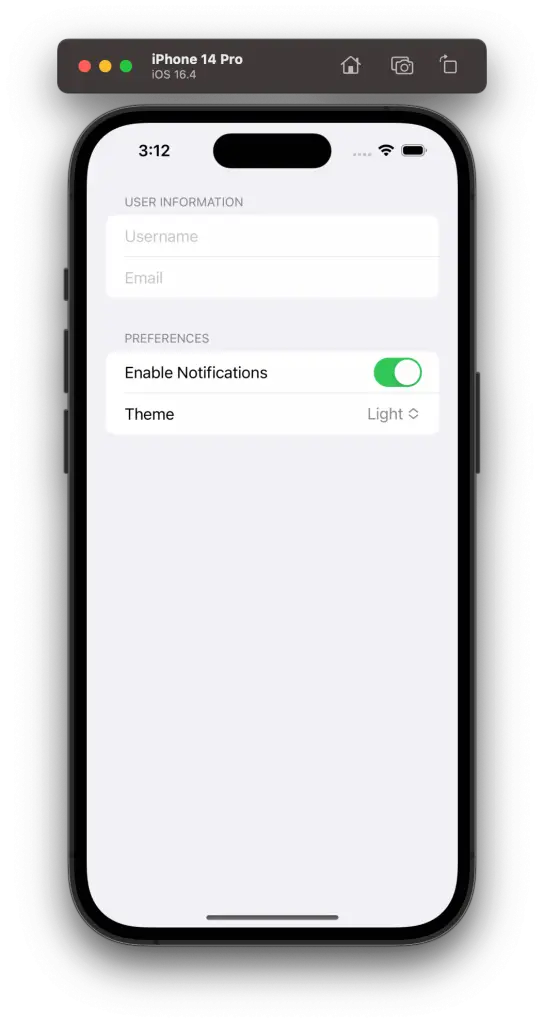
Explanation:
Section(header: Text("Title"))
: Adds a title to a specific section of the Form. You can add different titles to different sections to organize your Form.
Add Navigation Bar Title
The navigation bar title gives an overall context to the form, helping users understand the purpose of the page. Here’s an example:
import SwiftUI
struct ContentView: View {
@State private var username = ""
@State private var email = ""
var body: some View {
NavigationView {
Form {
Section(header: Text("User Information")) {
TextField("Username", text: $username)
TextField("Email", text: $email)
}
Section(header: Text("Preferences")) {
Toggle("Enable Notifications", isOn: .constant(true))
Picker("Theme", selection: .constant(1)) {
Text("Light").tag(1)
Text("Dark").tag(2)
}
}
}
.navigationBarTitle("Settings", displayMode: .inline)
}
}
}
Explanation:
.navigationBarTitle("Settings", displayMode: .inline)
: This modifier adds a title to the navigation bar. ThedisplayMode
parameter can be.inline
,.large
, or.automatic
, allowing control over the appearance.
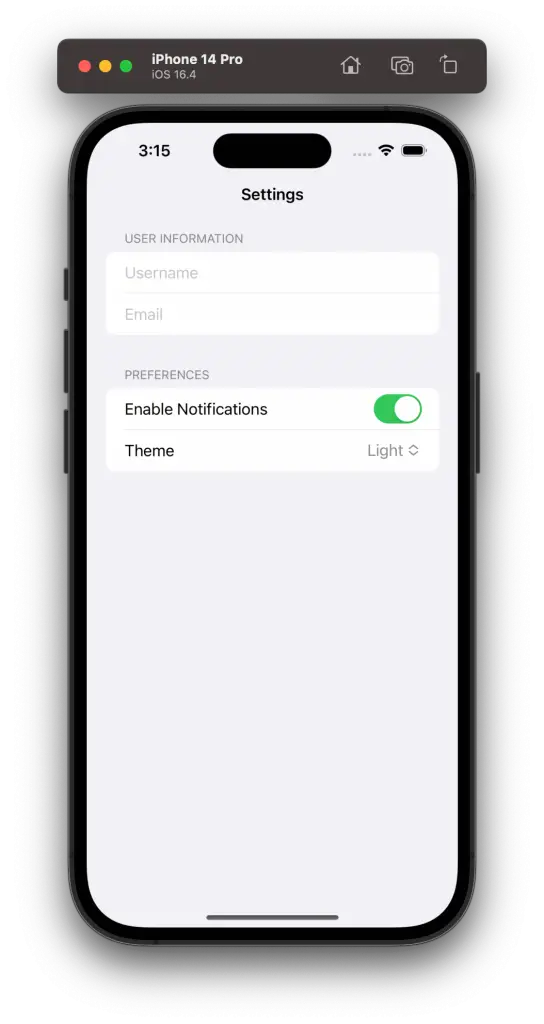
Tips for Effective Title Usage
Consider Length:
- Keep It Short: A concise title is often more effective. It conveys the purpose without overwhelming the user.
Maintain Consistency:
- Uniform Style: Use consistent font styles and sizes across different titles for a cohesive look.
Accessibility:
- Support for VoiceOver: Make sure the titles are accessible to VoiceOver users by testing them with accessibility tools.
Titles are a powerful tool in SwiftUI to enhance the user’s navigation experience within Forms. By understanding how to use section titles and navigation bar titles effectively, you can make your Forms more organized, visually appealing, and user-friendly.
Remember to keep the titles concise, maintain uniformity, and ensure accessibility to make the most of this feature.