How to Create a Half Screen Sheet in iOS SwiftUI
In SwiftUI, sheets are a popular way to present secondary views or modals. While SwiftUI does a good job of automatically sizing these sheets, there might be times when you want a sheet to take up only half of the screen.
In this blog post, we’ll explore how to create a half-screen sheet in SwiftUI using the .presentationDetents modifier with the .medium option.
Create a Half-Screen Sheet
Example Code
import SwiftUI
struct ContentView: View {
@State private var showModal = false
var body: some View {
Button("Show Modal") {
showModal.toggle()
}
.sheet(isPresented: $showModal) {
VStack{
Text("Hello, I'm a modal!")
Button("Dismiss") {
showModal = false
}
}.presentationDetents([.medium])
}
}
}
Code Explanation
.presentationDetents([.medium])
: The.presentationDetents
modifier allows you to control the size of the sheet. The.medium
option sets the sheet to take up half of the screen.Button("Show Modal")
: This button toggles theshowModal
state variable, which controls the presentation of the sheet.Button("Dismiss")
: Inside the sheet, we have a button that setsshowModal
tofalse
, effectively dismissing the sheet.
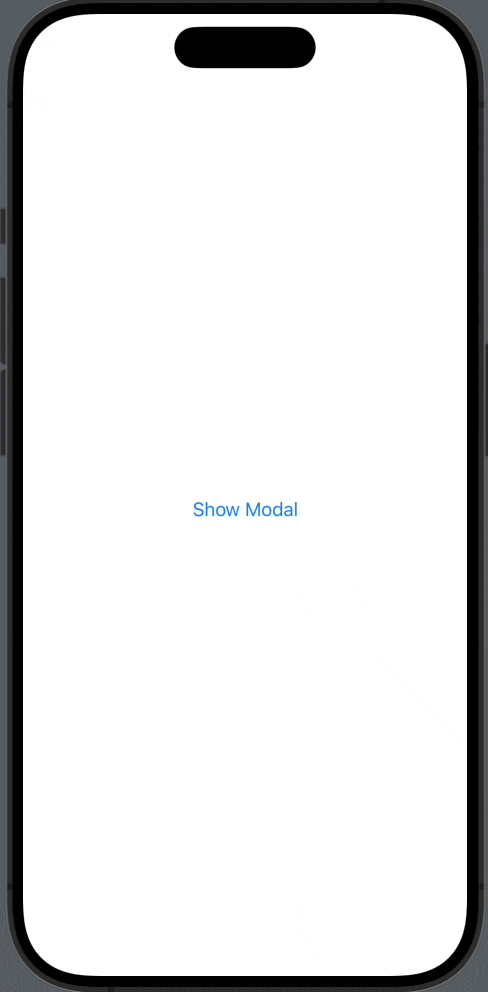
Why Use a Half-Screen Sheet?
Here are some reasons why you might want to use a half-screen sheet:
- User Experience: A half-screen sheet can be less intrusive and allows the user to maintain some context of the underlying view.
- Design Consistency: If your design guidelines specify certain sizes for modals, a half-screen sheet can help maintain that consistency.
- Content Suitability: For content that doesn’t require a full-screen view, a half-screen sheet can be more appropriate.
Creating a half-screen sheet in SwiftUI is straightforward with the .presentationDetents
modifier. The .medium
option allows you to easily set the sheet to take up half of the screen, providing a more flexible and user-friendly way to present secondary views or modals in your app.
One Comment