How to Change Toggle Background Color in iOS SwiftUI
Toggles are important components in mobile app development, allowing users to switch between two states. While SwiftUI provides a default look for toggles, you might want to customize them to better fit your app’s design. One common customization is changing the background color.
In this blog post, we’ll explore how to change the background color of a toggle in SwiftUI.
The Default Toggle
First, let’s start with a basic toggle. The code to create one is simple:
import SwiftUI
struct ContentView: View {
@State private var isOn = true
var body: some View {
Toggle("Enable Feature", isOn: $isOn).padding()
}
}
This will create a toggle with the default background color.
Use Background Modifier
The simplest way to change the background color is by using the background
modifier:
import SwiftUI
struct ContentView: View {
@State private var isOn = true
var body: some View {
Toggle("Enable Feature", isOn: $isOn).padding().background(Color.gray)
}
}
This will change the background color to gray, but it will cover the entire toggle, not just the switch.
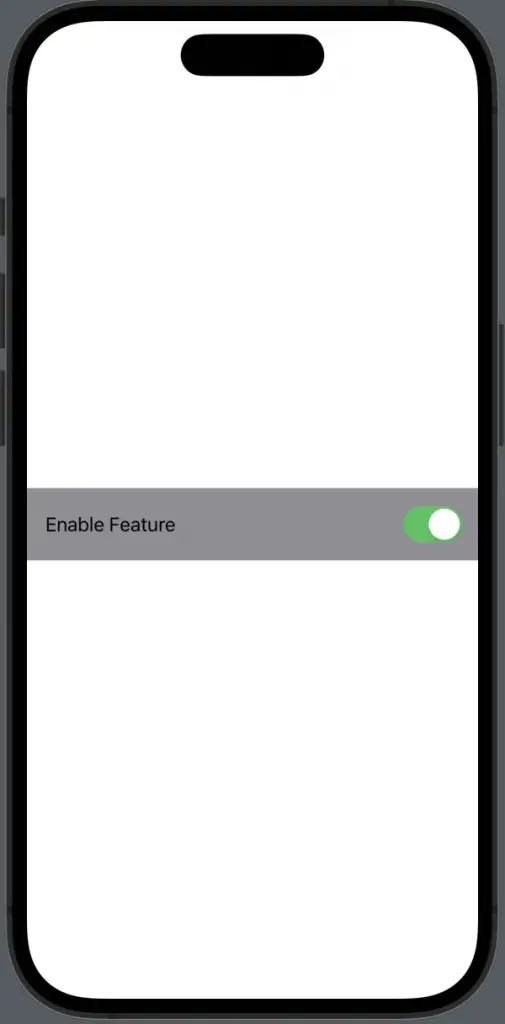
Conditional Background Color
You can also set the background color conditionally based on the toggle’s state:
import SwiftUI
struct ContentView: View {
@State private var isOn = true
var body: some View {
Toggle("Enable Feature", isOn: $isOn).padding().background(isOn ? Color.gray : Color.red)
}
}
This will set the background to green when the toggle is on and red when it’s off.
Changing the background color of a toggle in SwiftUI can be done in various ways. You can use the background
modifier for a quick change, create a custom toggle style for full control, or set the background color conditionally. Choose the method that best suits your app’s needs.