How to Adjust Image Opacity in Android Jetpack Compose
Adjusting the opacity of an image can be a crucial part of user interface design. It helps in distinguishing UI elements and adds an aesthetic touch to your application. In this updated blog post, we’ll dive deeper into how you can modify image opacity using Android Jetpack Compose’s Image
composable.
Opacity and Alpha Parameter
Opacity is essentially how “see-through” a given UI element is. In Android Jetpack Compose, the alpha
parameter allows us to set the opacity level for images. A value of 1 means the image is fully opaque, while 0 makes it entirely transparent.
Code Example: Set Image Opacity
Jetpack Compose makes it super easy to change the opacity of an image. Below is the code snippet where we set the alpha
parameter to adjust opacity.
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = null,
alpha = 0.5f
)
}
In this example, we’ve used an image resource called pet
and set its opacity to 50%. Make sure that the image is added to Android Studio.
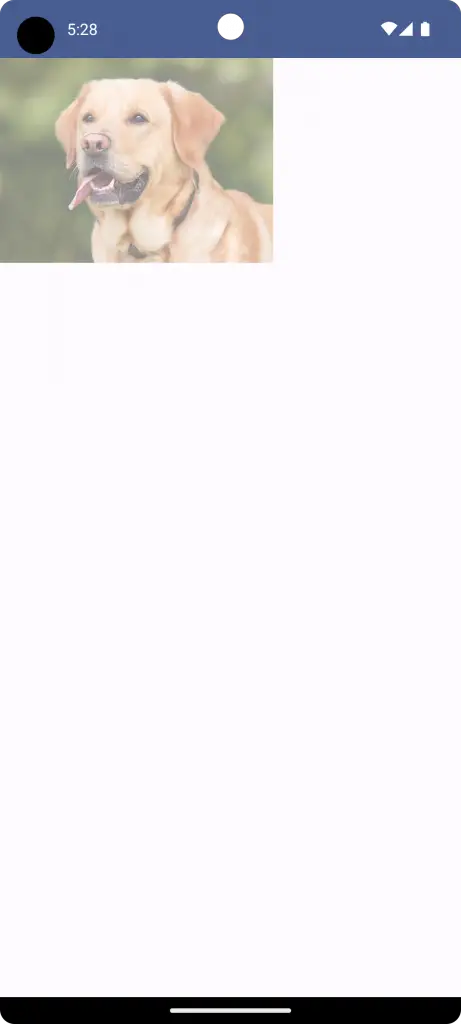
Complete Code Example: Jetpack Compose Image Opacity
Below is the complete Kotlin code that demonstrates how to implement image opacity in Jetpack Compose.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ImageExample()
}
}
}
}
}
}
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = null,
alpha = 0.5f
)
}
The MainActivity
class sets the content to our ImageExample
composable function, displaying an image with 50% opacity.
Adjusting the opacity of an image in Jetpack Compose is straightforward. Just use the alpha
parameter in the Image
composable. You can set it to any value between 0 and 1 to get your desired level of opacity. Play around with it to make your UI more interactive and visually appealing.
One Comment