How to Add Chip in Android Jetpack Compose
Chips are compact elements that display a piece of information, action, or attribute. In Jetpack Compose, creating chips is straightforward and customizable. In this post, we’ll explore how to add a Filter Chip using Jetpack Compose and Kotlin.
Chip Example Code
Below is a code example that demonstrates how to add a Filter Chip in Jetpack Compose.
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ChipExample() {
var selected by remember { mutableStateOf(false) }
FilterChip(
onClick = { selected = !selected },
modifier = Modifier.padding(10.dp),
colors = FilterChipDefaults.filterChipColors(
containerColor = Color.Gray,
iconColor = Color.White,
labelColor = Color.White,
selectedContainerColor = Color.Cyan,
selectedLabelColor = Color.Black,
selectedLeadingIconColor = Color.Black
),
label = { Text("Filter Chip") },
selected = selected,
leadingIcon = {
Icon(
Icons.Filled.Check,
contentDescription = null
)
}
)
}
Code Breakdown
Let’s understand each segment of this code:
Opt-In for ExperimentalMaterial3Api
- We start by opting into the experimental Material3 API using
@OptIn(ExperimentalMaterial3Api::class)
.
Chip Composable Function
ChipExample
is a Composable function where we define our Filter Chip.
Mutable State
- The
selected
variable tracks whether the chip is selected or not.
FilterChip
- We use
FilterChip
to create the chip and set its attributes like colors, label, and icon.
Colors and Style
- The
colors
parameter lets you customize various aspects like container color, icon color, and label color.
Label and Leading Icon
- The
label
andleadingIcon
parameters let you add a text label and an icon to the chip.
Click Event
- The
onClick
parameter toggles theselected
state when you tap on the chip.
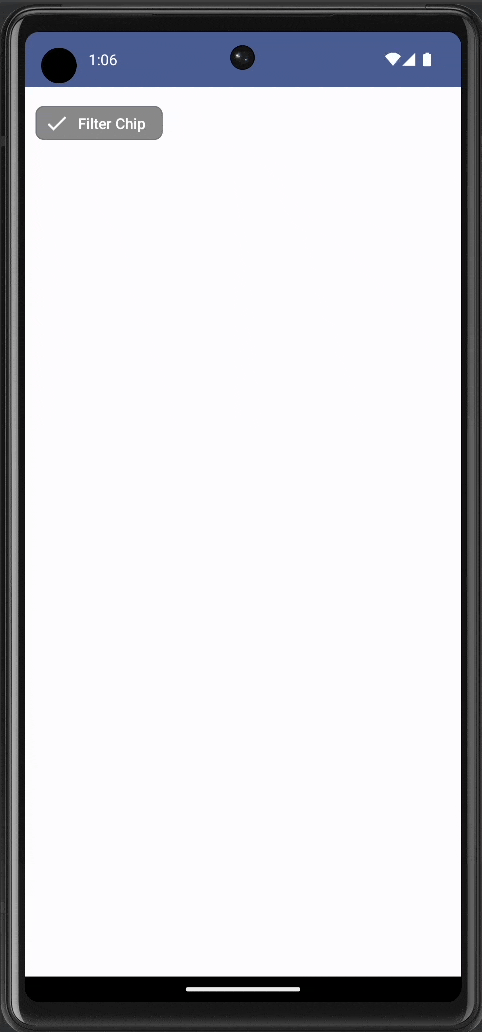
Complete Code
To use this chip in your app, add the ChipExample()
function inside your main Composable content. Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Check
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.FilterChip
import androidx.compose.material3.FilterChipDefaults
import androidx.compose.material3.Icon
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ChipExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun ChipExample() {
var selected by remember { mutableStateOf(false) }
FilterChip(
onClick = { selected = !selected },
modifier = Modifier.padding(10.dp),
colors = FilterChipDefaults.filterChipColors(containerColor = Color.Gray, iconColor = Color.White, labelColor = Color.White,
selectedContainerColor = Color.Cyan, selectedLabelColor = Color.Black, selectedLeadingIconColor = Color.Black),
label = {Text("Filter Chip")},
selected = selected,
leadingIcon = {
Icon(
Icons.Filled.Check,
contentDescription = null
)
}
)
}
Adding a chip in Jetpack Compose is relatively easy and offers lots of customization options. You can modify colors, add icons, and even track its selected state. It’s an excellent way to display compact, interactive information.