How to add Snackbar in Android Jetpack Compose
The Snackbar is a UI component to show brief messages with an option to do a specific action. It usually appears at the bottom of the screen and does not interrupt the user. Let’s learn how to create a simple Snackbar in Jetpack Compose.
The Snackbar in compose helps us to send a short message with action to users. See the following code snippet.
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Scaffold(
snackbarHost = { SnackbarHost(snackbarHostState) },
content = { innerPadding ->
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.padding(innerPadding)
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
}
)
}
In the above example:
We start by creating a remember { SnackbarHostState() } which maintains the Snackbar’s state across recompositions. We use rememberCoroutineScope() to launch a coroutine within the current composable scope. This is necessary because showSnackbar is a suspending function and hence needs to be called from within a coroutine.
We wrap the UI in a Scaffold composable function that provides a high-level structure for your screen and can host a SnackbarHost. The SnackbarHost is defined within the Scaffold and is provided the SnackbarHostState.
Inside the content lambda of Scaffold, we add a Button which, when clicked, initiates the process to show the Snackbar. When the button is clicked, we launch a coroutine and call snackbarHostState.showSnackbar.
This function takes several parameters: the message to be displayed, an optional action label, whether to include a dismissal action, and the duration for which the Snackbar should be displayed.
We store the result of showSnackbar in a variable. This function returns a SnackbarResult which can be either ActionPerformed or Dismissed. Based on the result, we perform the required action.
In this way, when the “Show Snackbar” button is clicked, a Snackbar will be shown with the message “Snackbar Example” and an “Action” button. The Snackbar will stay on the screen until the user interacts with it, either by pressing the action or by dismissing the Snackbar.
If the action button is clicked, or the Snackbar is dismissed, the respective blocks under the when condition is triggered, allowing you to define custom behavior.
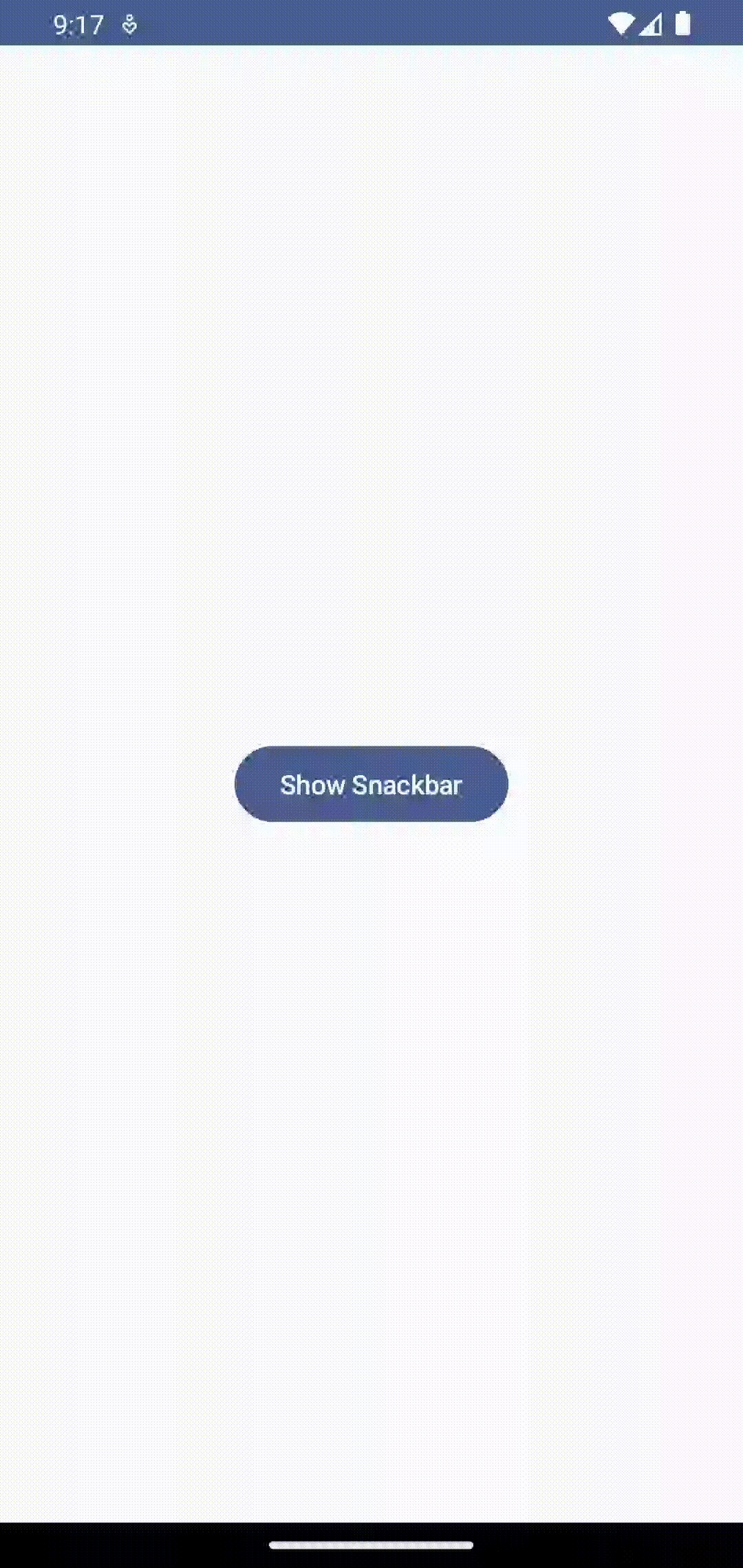
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.wrapContentSize
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.SnackbarDuration
import androidx.compose.material3.SnackbarHost
import androidx.compose.material3.SnackbarHostState
import androidx.compose.material3.SnackbarResult
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.runtime.rememberCoroutineScope
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
import kotlinx.coroutines.launch
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SnackbarExample()
}
}
}
}
}
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Scaffold(
snackbarHost = { SnackbarHost(snackbarHostState) },
content = { innerPadding ->
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.padding(innerPadding)
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
}
)
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SnackbarExample()
}
}
You can also create Snackbar without using Scaffold too. With Jetpack Compose, adding a Snackbar to your Android application is quite straightforward. This new toolkit allows for more intuitive and interactive application development, so keep exploring its features.
One Comment