How to Enable TextField Auto Correct in Android Jetpack Compose
The auto-correct feature is often handy for long-form typing, but it can become a nuisance when entering short texts like usernames or names. In this tutorial, we’ll explore how to easily enable or disable the auto-correct feature in Android Jetpack Compose.
What is Android Jetpack Compose?
Jetpack Compose is a modern Android UI toolkit. It allows developers to build native Android interfaces more easily and quickly. If you haven’t used Jetpack Compose before, consider this article a quick starter guide for working with TextFields and auto-correct.
Enable TextField Auto Correct
First, let’s dive into the code. We’ll use the KeyboardOptions
class in association with TextField
to manipulate the auto-correct feature. See the following code snippet.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Sample Text") },
keyboardOptions = KeyboardOptions(autoCorrect = true),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
Complete Code
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Sample Text") },
keyboardOptions = KeyboardOptions(autoCorrect = true),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
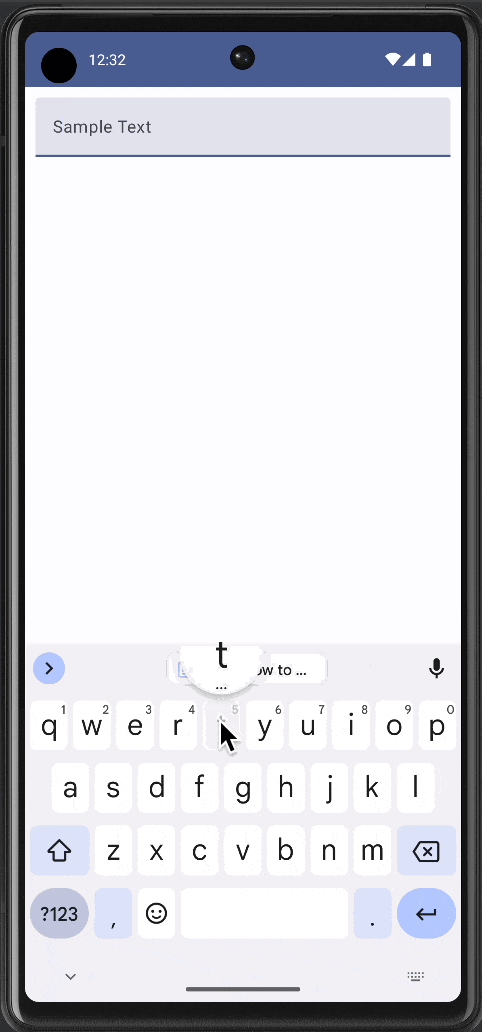
In this example, the KeyboardOptions
class enables auto-correct by setting autoCorrect = true
. When you use this option, your TextField component will automatically correct the text as you type.
So, there you have it! You now know how to enable or disable the auto-correct feature in TextFields using Android Jetpack Compose. You can fine-tune this setting according to the requirements of your application, making the user experience more flexible.