How to Add Hint Text to TextField in Android Jetpack Compose
Adding hint text to a TextField can significantly improve user experience. It guides users on what they need to enter in the input field. In this enhanced tutorial, we’ll cover how to add hint text or placeholder text to a TextField in Android Jetpack Compose, step by step.
What Is Hint Text and Why It Matters
Hint text is the placeholder text displayed in an input field before the user starts typing. It provides valuable cues to users, making the app’s UI more intuitive. Ignoring this small detail can lead to user confusion, so it’s good practice to include hint text.
Setting Up the TextField with Placeholder Parameter
In a previous blog post, I discussed adding a TextField in Jetpack Compose. The TextField composable has a parameter named placeholder
that makes adding hint text straightforward.
Here’s a code snippet that demonstrates this:
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
placeholder = { Text("Enter text") },
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
In this example, the placeholder text is “Enter text”.
Complete Code Example
Now let’s see how this fits into a complete Jetpack Compose example:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput, onValueChange = {textInput = it},
placeholder = { Text("Enter text") },
modifier = Modifier
.fillMaxWidth()
.padding(10.dp))
}
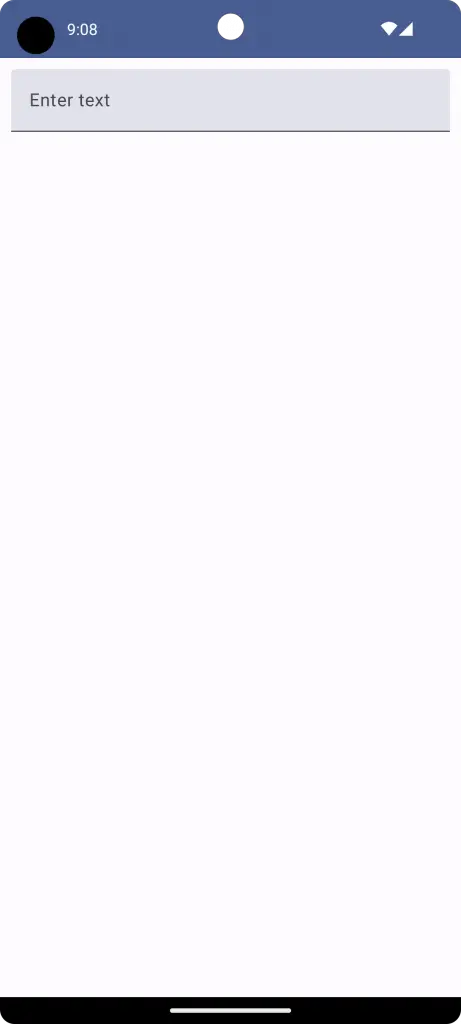
To sum up, adding hint text to a TextField is simple but adds much-needed clarity to your app. Always use the placeholder
parameter in the TextField
composable for this purpose. Choose the hint text carefully to offer clear instructions to users.
By following these practices, you’ll make your app more user-friendly and intuitive. Don’t overlook this small but crucial aspect of app design.
3 Comments