How to Draw Gradients Using Canvas in Android Jetpack Compose
Gradients are used to create subtle transitions between colors or to create striking and dynamic visual effects. In this blog post, let’s learn how to create simple gradients using Canvas in Jetpack Compose.
See the following code snippet.
Canvas(modifier = Modifier.fillMaxSize()) {
val brush = Brush.horizontalGradient(
colors = listOf(Color.Cyan, Color.Green),
)
drawRect(
brush = brush,
size = size
)
}
This code creates a Canvas component using the Canvas function from Jetpack Compose. The modifier parameter is set to Modifier.fillMaxSize(), which makes the Canvas fill the maximum available space in its parent layout.
Inside the Canvas, it declares a variable brush and assigns it to a Brush object created using the horizontalGradient function provided by the Brush class.
Then, it calls the drawRect function provided by the Canvas class. This function is used to draw a rectangle on the Canvas. It takes two parameters:
brush: The brush used to paint the rectangle, in this case, the brush variable which is assigned to the horizontal gradient brush created above.
size: The size of the rectangle represented by a Size object. The size property is set to size, which is the size of the Canvas component.
So, this code will draw a rectangle on the Canvas component, filling the entire space of the component with a horizontal gradient of two colors: Cyan and Green.
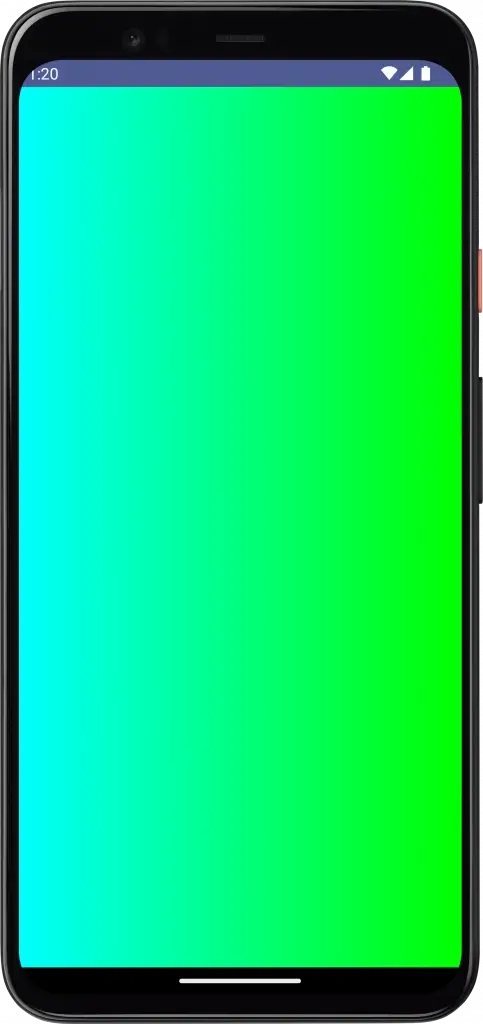
The horizontalGradient function creates a linear gradient brush that paints in a horizontal direction, this can be changed by using other variants of the Brush class such as verticalGradient or radialGradient which paints the gradient in a vertical or radial direction respectively.
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val brush = Brush.horizontalGradient(
colors = listOf(Color.Cyan, Color.Green),
)
drawRect(
brush = brush,
size = size
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw gradients using Canvas in Jetpack Compose.