How to Draw an Arc Using Canvas in Android Jetpack Compose
The Canvas in Jetpack Compose provides a powerful way to create and manipulate 2D graphics in your Android app. Specifically, its drawArc function is an essential tool for drawing arcs with precision.
In this blog post, let’s learn how to draw an arc easily using Canvas in Jetpack Compose. See the code snippet given below.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawArc(
Color.Blue,
topLeft = Offset(x = canvasWidth / 3f, y = canvasHeight / 3f),
startAngle = 30f,
sweepAngle = 120f,
useCenter = true,
size = Size(300F, 300F)
)
}
Here, we use the drawArc function provided by the Canvas. This function is used to draw an arc on the Canvas and we use its following parameters.
- color: The color of the arc, set to Color.Blue.
- topLeft: The top left corner of the arc represented by an Offset object. The x and y properties of the Offset are set to canvasWidth / 3f and canvasHeight / 3f respectively, which positions the arc’s starting point at the top left corner of the canvas and it will be 1/3rd from the left side and 1/3rd from the top side.
- startAngle: The starting angle of the arc in degrees. It is set to 30f.
- sweepAngle: The sweep angle of the arc in degrees. It is set to 120f.
- useCenter: It’s used to determine whether the arc should be drawn with a round end cap or a flat end cap. If set to true, the arc will be drawn with a round end cap.
- size: The size of the arc represented by a Size object. The height and width properties of the Size are set to 300F and 300F respectively, which defines the height and width of the arc as 300F.
You will get the following output.
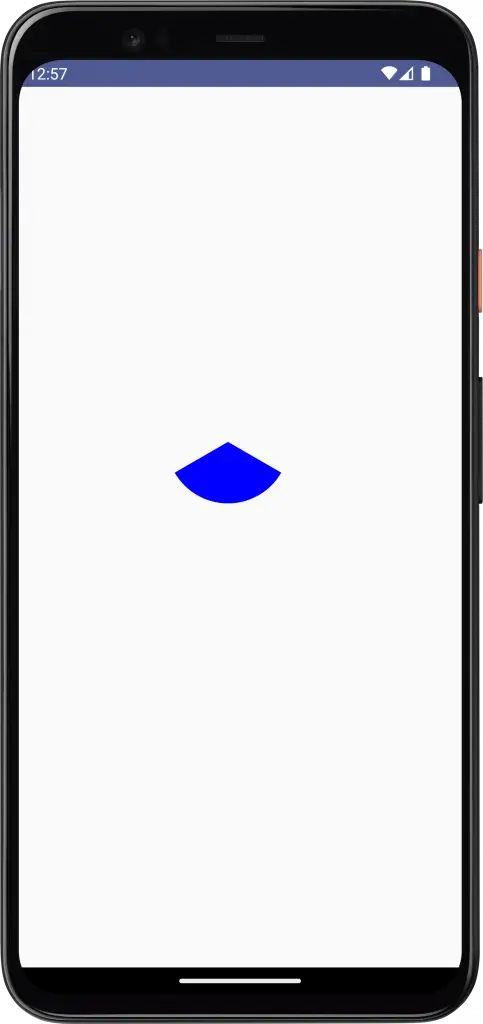
Following is the complete for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.geometry.Size
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawArc(
Color.Blue,
topLeft = Offset(x = canvasWidth / 3f, y = canvasHeight / 3f),
startAngle = 30f,
sweepAngle = 120f,
useCenter = true,
size = Size(300F, 300F)
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw an arc using Canvas in Jetpack Compose.
One Comment