How to Draw Oval Shape Using Canvas in Android Jetpack Compose
Canvas is a powerful tool that allows developers to create and manipulate 2D graphics in their Android apps. It provides a surface on which to perform drawing operations, such as drawing shapes, lines, and images.
In this blog post, let’s learn how to draw an oval using Canvas in Jetpack Compose. See the code snippet given below.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawOval(
color = Color.Red,
topLeft = Offset(x = canvasWidth / 4, y = canvasHeight / 3),
size = Size(height = 300F, width = 500F),
)
}
We the drawOval function provided by Canvas. This function is used to draw an oval shape on the Canvas. Here we used the following parameters.
- color: The color of the oval, set to Color.Red.
- topLeft: The top left corner of the oval is represented by an Offset object. The x and y properties of the Offset are set to canvasWidth / 4 and canvasHeight / 3 respectively, which positions the oval’s starting point at the top left corner of the canvas and it will be 1/4th from the left side and 1/3rd from the top side.
- size: The size of the oval represented by a Size object. The height and width properties of the Size are set to 300F and 500F respectively, which defines the height and width of the oval as 300F and 500F.
You will get the following output.
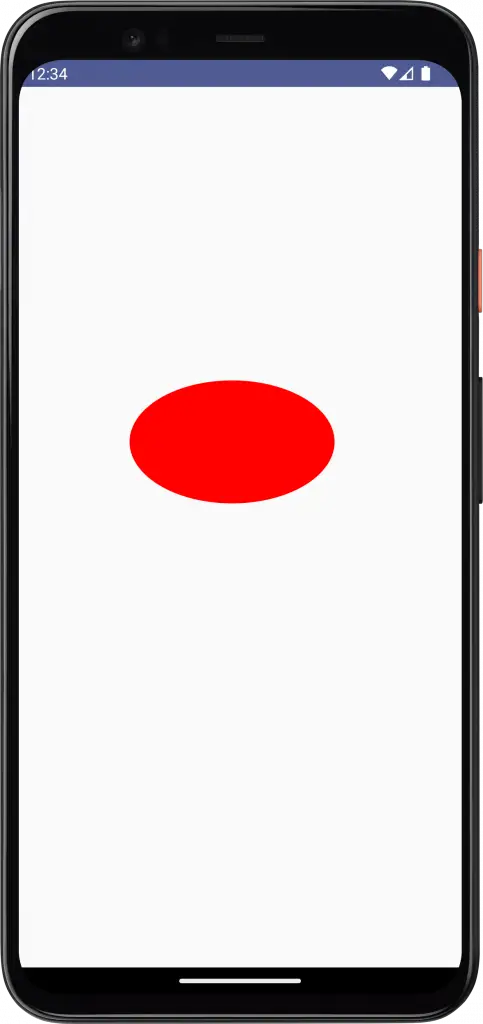
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.geometry.Size
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawOval(
color = Color.Red,
topLeft = Offset(x = canvasWidth / 4, y = canvasHeight / 3),
size = Size(height = 300F, width = 500F),
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw oval shapes using Canvas in Jetpack Compose.
One Comment