How to Draw a Rounded Rectangle Using Canvas in Android Jetpack Compose
In this blog post, let’s explore the capabilities of the Canvas in Jetpack Compose, and how you draw a rectangle with rounded corners using Canvas. We’ll be taking a look at the drawRoundRect function that allows you to draw rounded rectangles on the Canvas with ease.
See the following code snippet.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawRoundRect(
color = Color.Red,
topLeft = Offset(x = canvasWidth / 4, y = canvasHeight / 4),
size = Size(height = 500F, width = 500F),
cornerRadius = CornerRadius(x = 50f, y = 50f),
)
}
Inside the Canvas, we declare two variables canvasWidth and canvasHeight, and assign them to the width and height of the Canvas. These values can be used later to position elements on the Canvas.
Then, we call the drawRoundRect function provided by Canvas. This function is used to draw a rounded rectangle on the Canvas and here we use its four parameters.
- color: The color of the rectangle is set to Color.Red.
- topLeft: The top left corner of the rectangle represented by an Offset object. The x and y properties of the Offset are set to canvasWidth / 4 and canvasHeight / 4 respectively, which positions the rectangle’s starting point at the top left corner of the canvas and it will be 1/4th from the left side and 1/4th from the top side.
- size: The size of the rectangle represented by a Size object. The height and width properties of the Size are set to 500F and 500F respectively, which defines the height and width of the rectangle as 500F.
- cornerRadius: The radius of the rectangle’s corner represented by the CornerRadius object. The x and y properties of the CornerRadius are set to 50f and 50f respectively, which defines the horizontal and vertical radius of the rounded corner of the rectangle.
Following is the output.
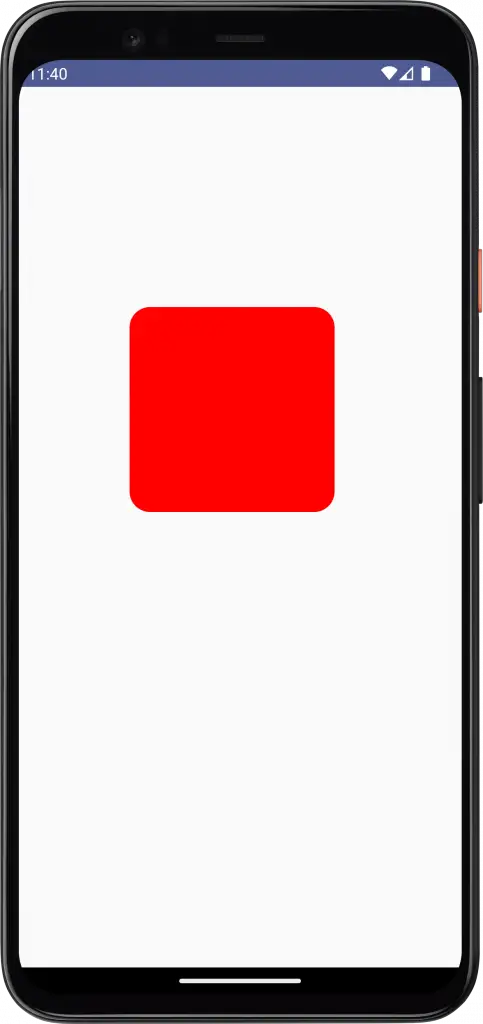
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.CornerRadius
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.geometry.Size
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawRoundRect(
color = Color.Red,
topLeft = Offset(x = canvasWidth / 4, y = canvasHeight / 4),
size = Size(height = 500F, width = 500F),
cornerRadius = CornerRadius(x = 50f, y = 50f),
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw a rectangle with rounded corners using Canvas in Jetpack Compose.