How to Get Screen Orientation in Android Jetpack Compose
Jetpack Compose is revolutionizing the way we build UI for Android applications. Its modern declarative style makes it easier for developers to handle UI states. This article will guide you on how to get the screen orientation in Android Jetpack Compose.
Screen orientation refers to the rotation of the screen from the natural portrait to landscape mode, and vice versa. This change often triggers different configurations in Android, like different layouts for portrait and landscape modes.
Jetpack Compose does not provide an explicit way to check the screen orientation. However, we can get the orientation by comparing the height and width of the screen. We make use of the LocalConfiguration Composable to get the current configuration of the system.
Here is an example of how to do it:
@Composable
fun ScreenOrientationExample() {
val configuration = LocalConfiguration.current
val orientation = if (configuration.orientation == Configuration.ORIENTATION_LANDSCAPE) {
"Landscape"
} else {
"Portrait"
}
Text("Screen orientation is: $orientation")
}
In the above code, we first get the current configuration via LocalConfiguration.current. Then, we compare configuration.orientation with Configuration.ORIENTATION_LANDSCAPE to determine whether the screen is in landscape mode. If it’s not in landscape, we assume it’s in portrait mode.
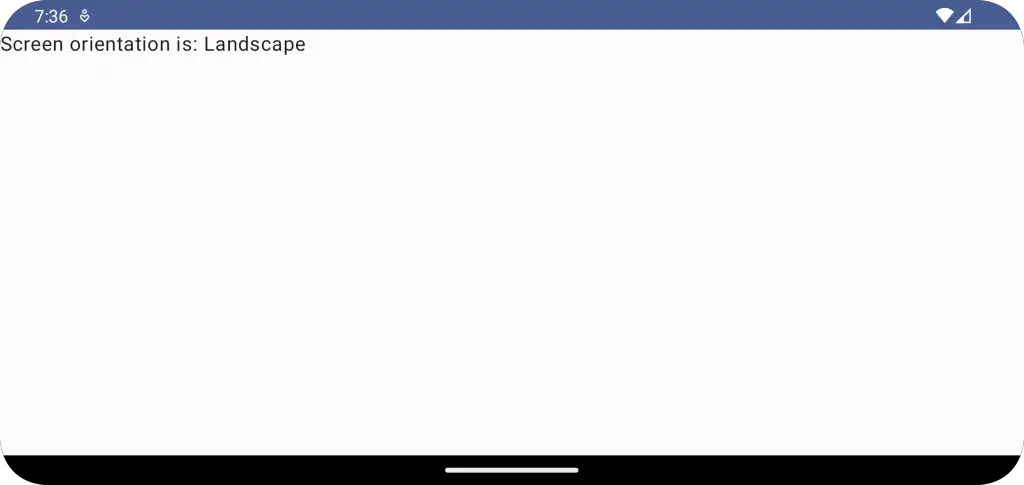
Having access to the screen orientation can be useful for adjusting your UI based on the orientation. This could mean showing or hiding certain elements, changing the layout entirely, or perhaps just making minor adjustments to padding or spacing.