How to show Text over an Image in Android Jetpack Compose
Nowadays, Android developers prefer Jetpack Compose toolkit for creating the UI of their apps. I already have a tutorial on adding images and in this blog post, let’s learn how to show text over an Image in Jetpack Compose.
First, you need to load the image you want to use as an overlay. In Jetpack Compose, you can use the Image component to load an image from a resource, a file, or a URL. Once the image is loaded, you can use it as a background for your overlay.
Next, you can add text on top of the image by using the Box composable. The Box is a container that can hold multiple child components and arrange them in a stack. In our case, we’ll use it to add Text on top of the Image.
To position the text on top of the image, we’ll use the Modifier’s align property. To move the Text component to the top of the Box, we’ll set the align property to Alignment.BottomCenter.
Here’s an example code snippet to demonstrate how to create an image overlay with text in Jetpack Compose.
@Composable
fun ImageExample() {
Box {
val image = painterResource(id = R.drawable.cake)
Image(painter = image, contentDescription = null)
Text(text = "Happy Birthday!", modifier = Modifier.align(Alignment.BottomCenter))
}
}
The Box container is created with no parameters inside the Composable function. This creates a layout container that can hold multiple child components and arrange them in a stack.
A variable called image is declared and assigned a value using the painterResource method. This method loads an image from a drawable resource in the app’s resources directory. In this example, the image is loaded from a drawable with the ID R.drawable.cake.
The Image component is added as a child component inside the Box container. The painter parameter of the Image component is set to the image variable that was defined earlier, which loads the image from the drawable resource.
A Text component is added as a child component inside the Box container. The text parameter of the Text component is set to “Happy Birthday!”.
The modifier parameter of the Text component is set to Modifier.align(Alignment.BottomCenter), which positions the text at the bottom center of the Box container.
You will get the following output.
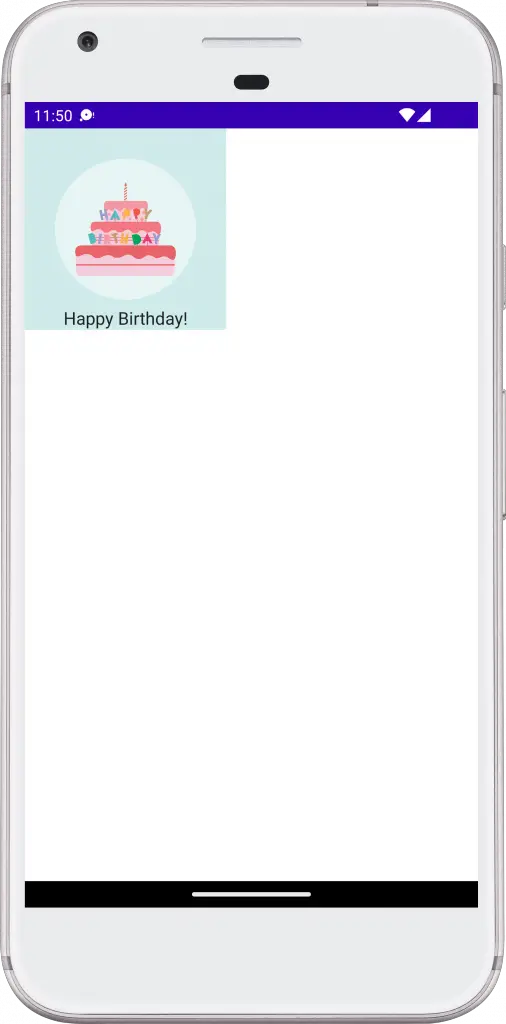
The complete code is given below for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Box {
val image = painterResource(id = R.drawable.cake)
Image(painter = image, contentDescription = null)
Text(text = "Happy Birthday!", modifier = Modifier.align(Alignment.BottomCenter))
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
That’s how you display text over an image in Jetpack Compose. I hope this Jetpack Compose tutorial to place text over image will be helpful for you.