How to Add Surface with Rounded Corners in Android Jetpack Compose
Android Jetpack Compose is a modern, fully declarative UI toolkit that is transforming the way developers build user interfaces on Android. It provides a simpler way to design and construct interfaces with less boilerplate code.
Among the many components it provides, Surface is a foundational one used to create containers with a specific appearance. In this tutorial, we will see how to create a Surface with rounded corners.
What is a Surface in Jetpack Compose
In Jetpack Compose, a Surface is a container that holds content and controls its layout details like padding, shape, background color, elevation, etc. It follows Material Design guidelines and often acts as a backdrop for higher-level composables. Surface is frequently used to modify the look and feel of the nested components.
How to Create Surface with Rounded Corners
Creating a rounded corner surface in Jetpack Compose is quite straightforward. You can use the RoundedCornerShape composable for this purpose. It allows you to define rounded corners for your surface.
Below is a simple example of how you can create a surface with rounded corners:
@Composable
fun RoundedCornerSurfaceExample() {
Surface(
shape = RoundedCornerShape(16.dp),
modifier = Modifier.padding(16.dp).fillMaxSize(),
color = Color.LightGray
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
In the code above, we have a Surface composable with a RoundedCornerShape applied to it, which gives the surface rounded corners. The 16.dp argument to RoundedCornerShape specifies the radius of the corners. Inside the Surface, we have a Text composable that simply displays a greeting message.
By running this code in your Composable function, you will see a grey surface with rounded corners containing the text “Hello, Jetpack Compose!”.
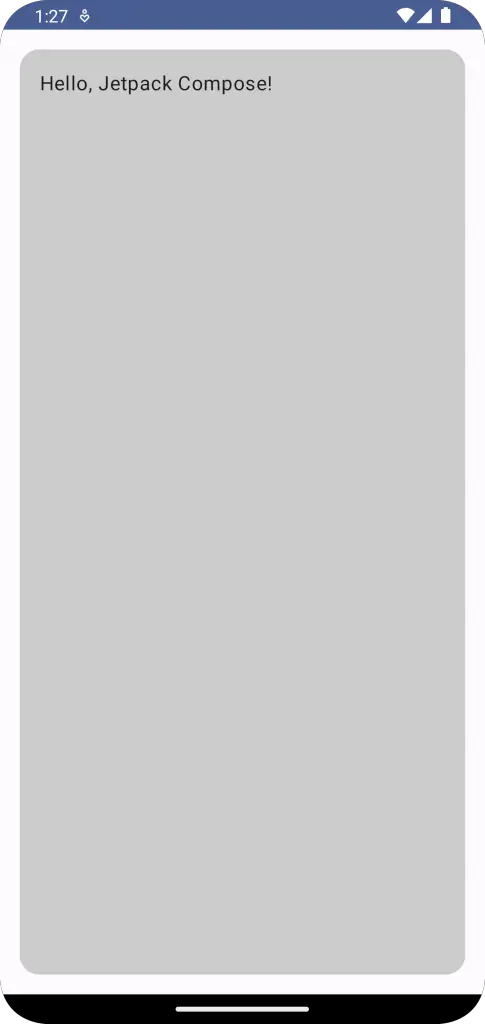
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
RoundedCornerSurfaceExample()
}
}
}
}
}
@Composable
fun RoundedCornerSurfaceExample() {
Surface(
shape = RoundedCornerShape(16.dp),
modifier = Modifier.padding(16.dp).fillMaxSize(),
color = Color.LightGray
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
RoundedCornerSurfaceExample()
}
}
In this blog post, we have learned how to create a Surface with rounded corners in Jetpack Compose. Jetpack Compose provides a flexible and intuitive way to create UIs. By understanding how to work with Surfaces, you can control the appearance of your containers more effectively and design beautiful Android apps.