How to Create Toast Message in Android Jetpack Compose
A toast message is a quick and simple way to give feedback to users in Android apps. You’ve probably seen these pop-up messages that fade away on their own after a few seconds. In this blog post, you’ll learn how to display a toast message in Android using Jetpack Compose.
Example Code: Toast in Jetpack Compose
Here’s a quick example to show a toast message using Jetpack Compose:
@Composable
fun ToastExample() {
val context = LocalContext.current
Button(onClick = { Toast.makeText(context, "This a simple toast tutorial!", Toast.LENGTH_LONG).show() }) {
Text("Click here to show toast!")
}
}
Code Breakdown
Let’s dissect the code snippet above to understand each component:
LocalContext
LocalContext
provides access to the context within a Composable.val context = LocalContext.current
grabs the current context.
Button Composable
- The
Button
Composable is used to create a clickable button. - The
onClick
event handler is triggered when the button is clicked.
Toast.makeText
- This is the standard Android method to make a toast.
- It takes three parameters:
context
: the application contexttext
: the message you want to showduration
: how long the toast should be displayed (Toast.LENGTH_SHORT
orToast.LENGTH_LONG
)
.show()
- This method is called to actually display the toast.
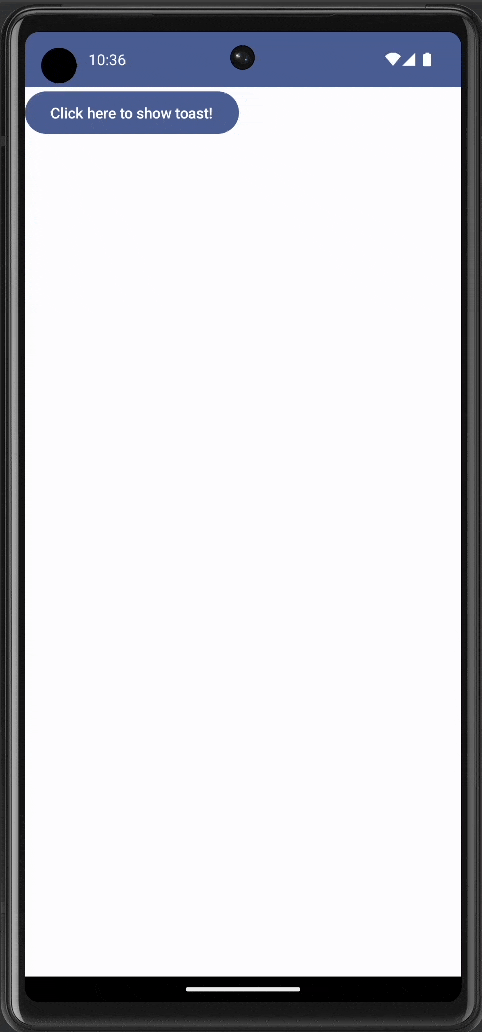
Integrate the Toast in Your App
To use this in your app, include the ToastExample()
Composable within your main activity’s content. Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import android.widget.Toast
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.platform.LocalContext
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ToastExample()
}
}
}
}
}
}
@Composable
fun ToastExample() {
val context = LocalContext.current
Button(onClick = { Toast.makeText(context,"This a simple toast tutorial!", Toast.LENGTH_LONG).show() }) {
Text("Click here to show toast!")
}
}
Displaying a toast message in Jetpack Compose is straightforward. By using the standard Android Toast
class inside a Composable, you can provide quick feedback to your users. Whether it’s for notifications, errors, or confirmations, toast messages are a handy tool in mobile app development.