How to Create Floating Action Button in Android Jetpack Compose
A Floating Action Button (FAB) is a key UI element in many Android applications. It’s a button that hovers over the UI, generally in the bottom corner, and provides quick access to a primary action. In this tutorial, we’ll guide you through the process of creating a FAB in Android using Jetpack Compose.
Why Use a FAB?
Before diving into the code, let’s understand why you might want to use a FAB. It’s designed to represent the primary action of an application. It grabs the user’s attention and is easy to reach.
Create a Simple Floating Action Button
Creating a FAB in Jetpack Compose is pretty straightforward. You can start by using the FloatingActionButton
component.
FloatingActionButton(onClick = { /*do something*/ }) {
Icon(Icons.Filled.Add, contentDescription = "Add Button")
}
This will create a simple FAB with an “Add” icon.
Place the FAB
Usually, FABs are placed at the bottom end area of the screen. To put your FAB in this location, you can use the following code snippet.
@Composable
fun FAB() {
Box(
modifier = Modifier.fillMaxSize().padding(20.dp),
){
Row(modifier = Modifier.align(Alignment.BottomEnd)){
FloatingActionButton(onClick = { /*do something*/ },) {
Icon(Icons.Filled.Add, contentDescription = "Add Button")
}
}
}
}
With this code, the FAB will show up at the bottom right corner of the screen.
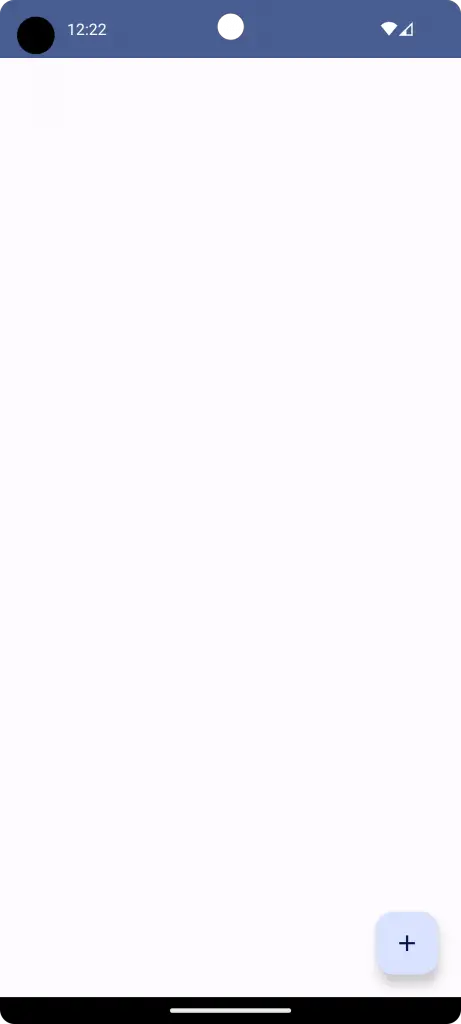
Complete Code
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material3.FloatingActionButton
import androidx.compose.material3.Icon
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
FAB()
}
}
}
}
}
@Composable
fun FAB() {
Box(
modifier = Modifier.fillMaxSize().padding(20.dp),
){
Row(modifier = Modifier.align(Alignment.BottomEnd)){
FloatingActionButton(onClick = { /*do something*/ },) {
Icon(Icons.Filled.Add, contentDescription = "Add Button")
}
}
}
}
Creating a Floating Action Button in Jetpack Compose is quite simple. It’s a great way to emphasize the main action your app is built around. By following this guide, you should be well on your way to incorporating this useful UI element into your Android apps.