How to Add Radio Button in Android Jetpack Compose
Radio buttons are essential UI elements that allow users to make a single selection from a list of options. This blog post will show you how to integrate radio buttons in your Android app using Jetpack Compose, Google’s modern Android UI toolkit.
The Radio Button Code Example
Here’s a Kotlin code example to help you create radio buttons in Jetpack Compose.
@Composable
fun RadioButtonExample() {
val options = listOf("Male", "Female", "Other")
val (selectedOption, onOptionSelected) = remember { mutableStateOf(options[0]) }
Column(Modifier.selectableGroup()) {
options.forEach { text ->
Row(
Modifier
.fillMaxWidth()
.selectable(
selected = (text == selectedOption),
onClick = { onOptionSelected(text) },
role = Role.RadioButton
)
.padding(8.dp),
) {
RadioButton(
selected = (text == selectedOption),
onClick = null
)
Text(
text = text,
modifier = Modifier.padding(start = 8.dp)
)
}
}
}
}
Code Explanation
Let’s dissect the code to understand each part:
State Variables
options
: A list of string values that serve as the choices for the radio buttons.selectedOption
: A state variable that holds the currently selected option.onOptionSelected
: A function that updates theselectedOption
.
Column and Row Composables
Column
: Arranges the radio button options vertically.Row
: Contains each radio button and its accompanying text.
Modifiers
Modifier.selectableGroup()
: Groups the radio button options together.Modifier.fillMaxWidth()
: Fills the available horizontal space.Modifier.padding(8.dp)
: Adds padding around each row.
RadioButton Composable
selected
: Specifies whether the radio button is selected.onClick
: Sets the click event. In this example, it’s set tonull
because the click event is handled by the Row.
Event Handling
onClick
withinselectable
: Updates theselectedOption
when a new radio button is selected.
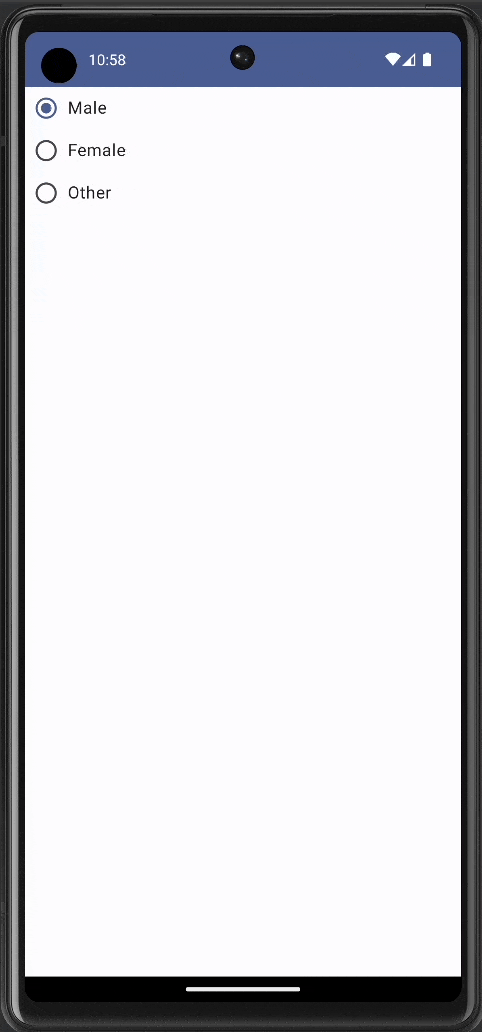
Incorporate Into Main Activity
To include this radio button example in your main Android project, simply add the RadioButtonExample()
function within your main activity’s content. Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.selection.selectable
import androidx.compose.foundation.selection.selectableGroup
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.RadioButton
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.semantics.Role
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
RadioButtonExample()
}
}
}
}
}
}
@Composable
fun RadioButtonExample() {
val options = listOf("Male", "Female", "Other")
val (selectedOption, onOptionSelected) = remember { mutableStateOf(options[0]) }
Column(Modifier.selectableGroup()) {
options.forEach { text ->
Row(
Modifier
.fillMaxWidth()
.selectable(
selected = (text == selectedOption),
onClick = { onOptionSelected(text) },
role = Role.RadioButton
)
.padding(8.dp),
) {
RadioButton(
selected = (text == selectedOption),
onClick = null
)
Text(
text = text,
modifier = Modifier.padding(start = 8.dp)
)
}
}
}
}
Adding radio buttons in Jetpack Compose is straightforward and flexible. You can also customize the radio button color, size, etc. With just a few lines of Kotlin code, you can create a fully functional radio button group for your Android application.