How to Add Slider in Android Jetpack Compose
Sliders are interactive UI components that allow users to select a value within a defined range. In this post, we will go through the steps to implement a slider in Jetpack Compose, Android’s modern UI toolkit.
Slider Example Code
Here’s a simple example to showcase how you can create a slider in Jetpack Compose:
@Composable
fun SliderExample() {
var position by remember { mutableStateOf(0f) }
Slider(
modifier = Modifier.padding(20.dp),
value = position,
onValueChange = { position = it },
valueRange = 0f..10f,
onValueChangeFinished = {
// do something
},
steps = 5,
)
}
Code Explanation
Let’s dissect the code and understand what each line does:
Declare State Variable
position
is a state variable that holds the current value of the slider.
Slider Composable
- The
Slider
Composable is used to create the slider. modifier = Modifier.padding(20.dp)
adds padding around the slider.
Value and Value Range
value = position
sets the current value of the slider.valueRange = 0f..10f
defines the range of the slider. In this example, it’s from 0 to 10.
Event Handlers
onValueChange = { position = it }
is called every time the slider value changes.onValueChangeFinished = { }
is called when the user releases the thumb.
Steps
steps = 5
divides the slider into 5 equal steps.
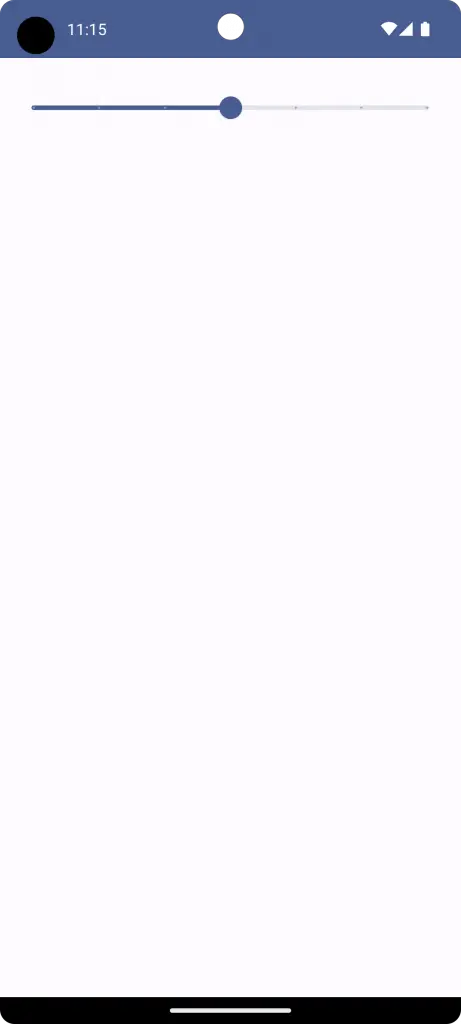
Incorporate Slider into Main Activity
To add this slider to your main activity, use the following code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Slider
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
SliderExample()
}
}
}
}
}
}
@Composable
fun SliderExample() {
var position by remember { mutableStateOf(0f) }
Slider(
modifier = Modifier.padding(20.dp),
value = position,
onValueChange = { position = it },
valueRange = 0f..10f,
onValueChangeFinished = {
// do something
},
steps = 5,
)
}
Implementing a slider in Jetpack Compose is both straightforward and versatile. You can easily customize it according to your needs and integrate it into your Android project.
This simple yet powerful UI component can enhance user interaction in various use-cases like volume control, brightness settings, or any range-based selection.
One Comment