How to Display Image in Black and White Color in Android Jetpack Compose
Displaying images in black and white can be a great way to add a touch of elegance or nostalgia to your app, and it’s easy to do using Jetpack Compose. In this blog post let’s learn how to show an image in black and white.
The Image composable has some useful parameters and the colorFilter is one among them. Combining the ColorFilter and the ColorMatrix class you can turn a color image into a black and white image.
You can also use ColorFilter to add tint color to images.
See the following code.
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix().apply { setToSaturation(0f) }),
modifier = Modifier.fillMaxSize()
)
By setting the saturation to zero, the image turns black and white.
Following is the image I used in this example.

See the following complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.aspectRatio
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix().apply { setToSaturation(0f) }),
modifier = Modifier.fillMaxSize()
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
You will get the following output.
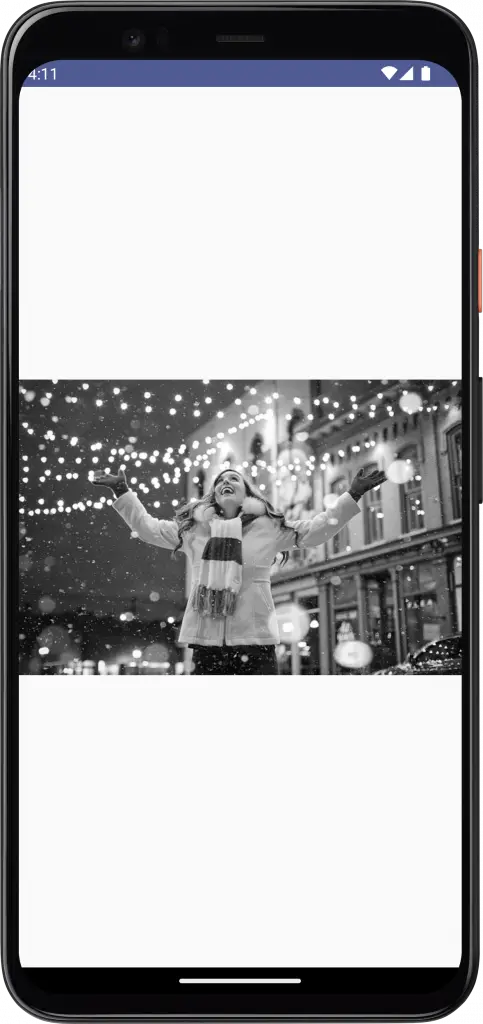
That’s how you show black and white images in Jetpack Compose.