How to Create Fade In and Fade Out Animations in Android Jetpack Compose
Animation is a powerful tool in the world of user interface design, adding visual interest and enhancing the overall user experience. It helps to make your app feel more dynamic and engaging. In this tutorial, let’s learn how to create a simple fade in and fade out animations in Jetpack Compose.
The APIs provided by Jetpack Compose are both powerful and flexible, allowing for the easy implementation of various animations within the user interface of your app. We use AnimatedVisibility API in this tutorial.
We can animate the appearance and disappearance using the AnimatedVisibility composable. The transition is customized using enter and exit parameters. See the following code snippet.
var visibility by remember { mutableStateOf(false) }
AnimatedVisibility(
visible = visibility,
enter = fadeIn(),
exit = fadeOut()
) {
Box(modifier = Modifier.size(100.dp).background(Color.Red))
}
We use the fadeIn function to create fade in transition and the fadeOut function to create fade out animation.
In the following example, we have a Button to toggle the visibility of the Box composable.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.AnimatedVisibility
import androidx.compose.animation.fadeIn
import androidx.compose.animation.fadeOut
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var visibility by remember { mutableStateOf(false) }
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { visibility = !visibility }) {
Text(text = "Click Here!")
}
AnimatedVisibility(
visible = visibility,
enter = fadeIn(),
exit = fadeOut()
) {
Box(modifier = Modifier.size(100.dp).background(Color.Red))
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
Following is the output.
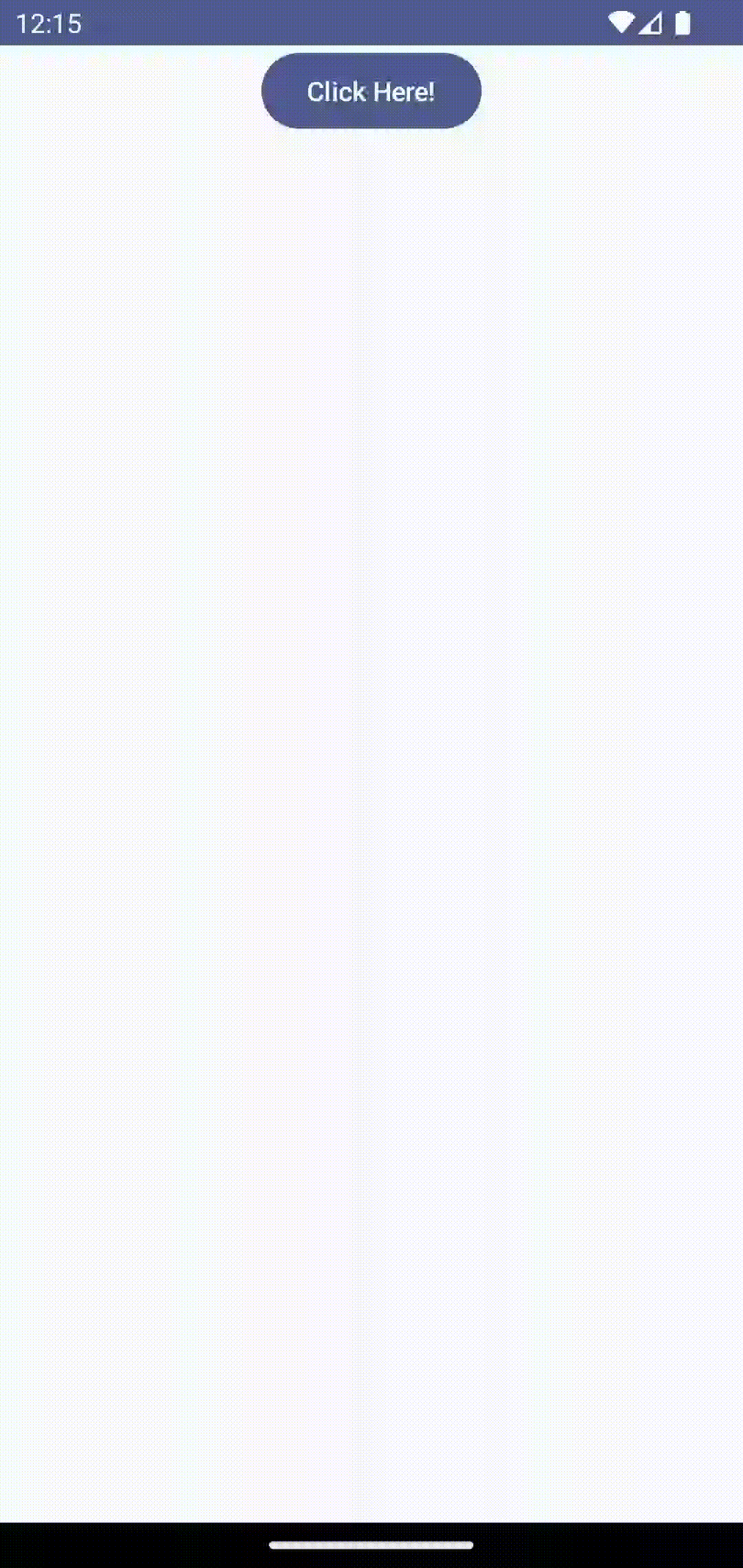
As you see, the fade in animation is there when the Box appears and fade out animation occurs at the time of disappearance.
That’s how you add fade in and fade out animations in react native.
One Comment