How to Make Column Clickable in Android Jetpack Compose
Column composable helps to arrange child content vertically in Jetpack Compose. Sometimes, you may want to make the Column clickable to add further functionality to the mobile app. Let’s learn how to make Column clickable easily in Jetpack Compose.
In Jetpack Compose, you can make composables clickable using Modifier.clickable{}. See the following code snippet where the Column is made clickable and a Toast message is shown when the Column is clicked.
@Composable
fun ColumnExample() {
val context = LocalContext.current
Column(modifier = Modifier
.fillMaxWidth()
.height(300.dp)
.padding(40.dp)
.background(Color.Cyan).clickable {
Toast
.makeText(context, " Clickable Column Example", Toast.LENGTH_SHORT)
.show()
})
{
Text(text = "Click here to test", fontSize = 24.sp)
}
}
You can also make Row clickable in the similar way.
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import android.widget.Toast
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ColumnExample()
}
}
}
}
}
}
@Composable
fun ColumnExample() {
val context = LocalContext.current
Column(modifier = Modifier
.fillMaxWidth()
.height(300.dp)
.padding(40.dp)
.background(Color.Cyan).clickable {
Toast
.makeText(context, " Clickable Column Example", Toast.LENGTH_SHORT)
.show()
})
{
Text(text = "Click here to test", fontSize = 24.sp)
}
}
The output is given below.
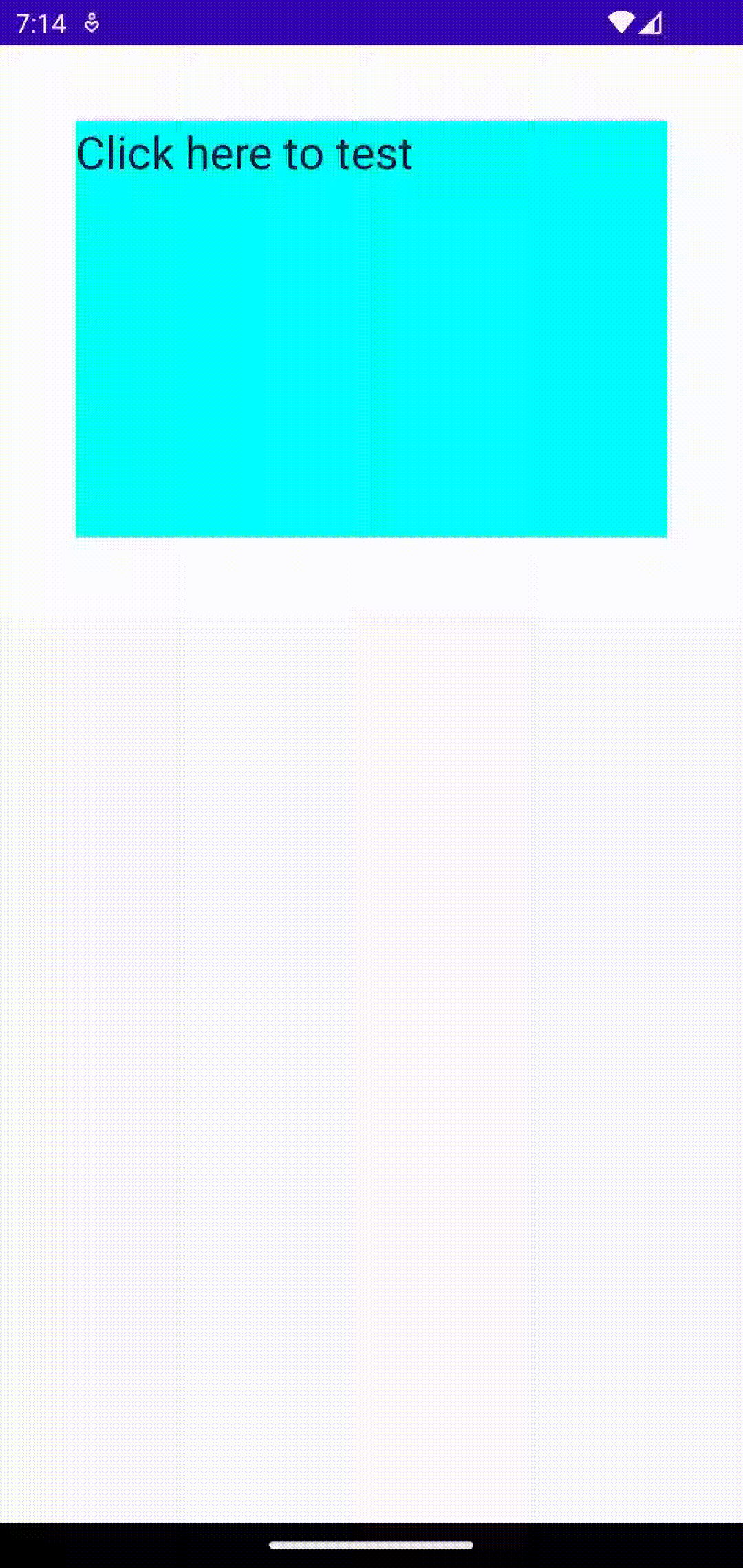
That’s how you make Column clickable in Jetpack Compose. I hope this Android tutorial is helpful for you.
One Comment