How to Add Space Between LazyColumn Items in Android Jetpack Compose
In Jetpack Compose, the LazyColumn is one of the most useful components. It allows you to efficiently display large lists of data. Sometimes, you might want to add some spacing between the items for a more aesthetic appearance or to separate different data more clearly.
In this tutorial, we’ll learn how to add space between items in a LazyColumn using the verticalArrangement attribute with Arrangement.spacedBy.
Arrangement.spacedBy
The Arrangement.spacedBy function is a predefined arrangement that places its children evenly with the specified spacing in-between. It’s a handy tool for managing space between elements in Jetpack Compose.
Add Space between Items in LazyColumn
The following example demonstrates how to add space between items in a LazyColumn using verticalArrangement = Arrangement.spacedBy(10.dp):
@Composable
fun SpacedItemList() {
val list = (1..100).toList()
LazyColumn(
verticalArrangement = Arrangement.spacedBy(10.dp),
modifier = Modifier.padding(16.dp)
) {
items(list) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
}
In this example, we’re creating a list from 1 to 100 and displaying it using a LazyColumn. We’re setting the verticalArrangement parameter to Arrangement.spacedBy(10.dp) to add 10 dp space between each item. Additionally, we’re using Modifier.padding(16.dp) to add padding around the whole LazyColumn.
When you run this example, you will notice that there is a space of 10dp between each item in the list, giving your UI a cleaner and more separated look.
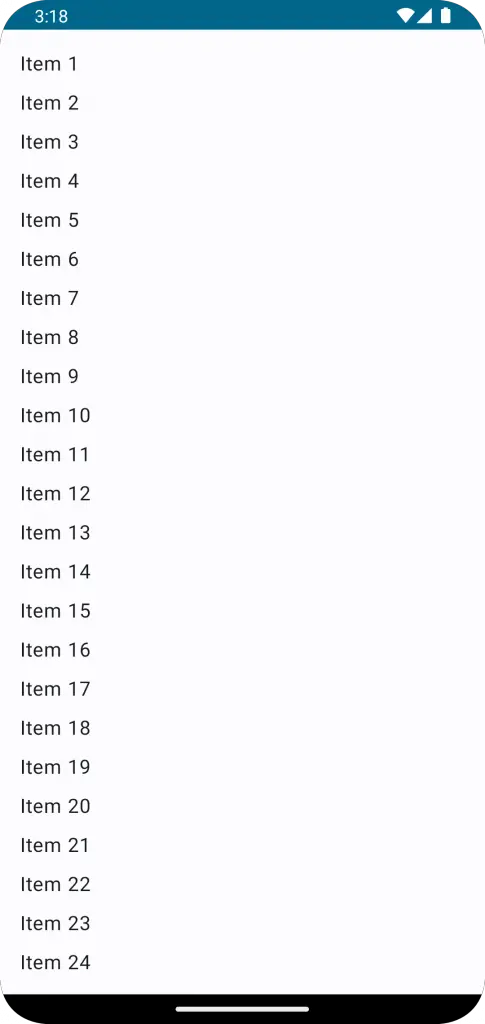
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.foundation.lazy.items
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SpacedItemList()
}
}
}
}
}
@Composable
fun SpacedItemList() {
val list = (1..100).toList()
LazyColumn(
verticalArrangement = Arrangement.spacedBy(10.dp),
modifier = Modifier.padding(16.dp)
) {
items(list) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SpacedItemList()
}
}
Remember, arranging elements in your user interface effectively can significantly impact the user experience of your app. Using Arrangement.spacedBy in LazyColumn is one of the many powerful tools Jetpack Compose provides to make this task easier.