How to Animate Color in Android Jetpack Compose
Animation is an important aspect that can increase the visual appeal of a mobile app. In this Android tutorial, let’s learn how to animate color in Jetpack Compose.
The Jetpack Compose provides both high level and low level APIs for animation. The animate*AsState functions are the simplest animation APIs for animating a single value. You can animate color using the animateColorAsState function.
See the code snippet given below.
var switch by remember { mutableStateOf(false) }
val bgColor: Color by animateColorAsState(if (switch) Color.Red else Color.Green)
Box(modifier = Modifier.size(100.dp).background(color = bgColor))
You can customize the animation using the animationSpec parameter.
var switch by remember { mutableStateOf(false) }
val bgColor: Color by animateColorAsState(if (switch) Color.Red else Color.Green,
animationSpec = tween(1000, easing = LinearEasing)
)
Box(modifier = Modifier.size(100.dp).background(color = bgColor))
Following is the complete example where I animate the background color of the Box composable. We have a button to initiate the animation which switches one color to another in the given time.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.animateColorAsState
import androidx.compose.animation.core.LinearEasing
import androidx.compose.animation.core.tween
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var switch by remember { mutableStateOf(false) }
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { switch = !switch }) {
Text(text = "Click Here!")
}
val bgColor: Color by animateColorAsState(if (switch) Color.Red else Color.Green,
animationSpec = tween(1000, easing = LinearEasing)
)
Box(modifier = Modifier.size(100.dp).background(color = bgColor))
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
Now, you will get the following output.
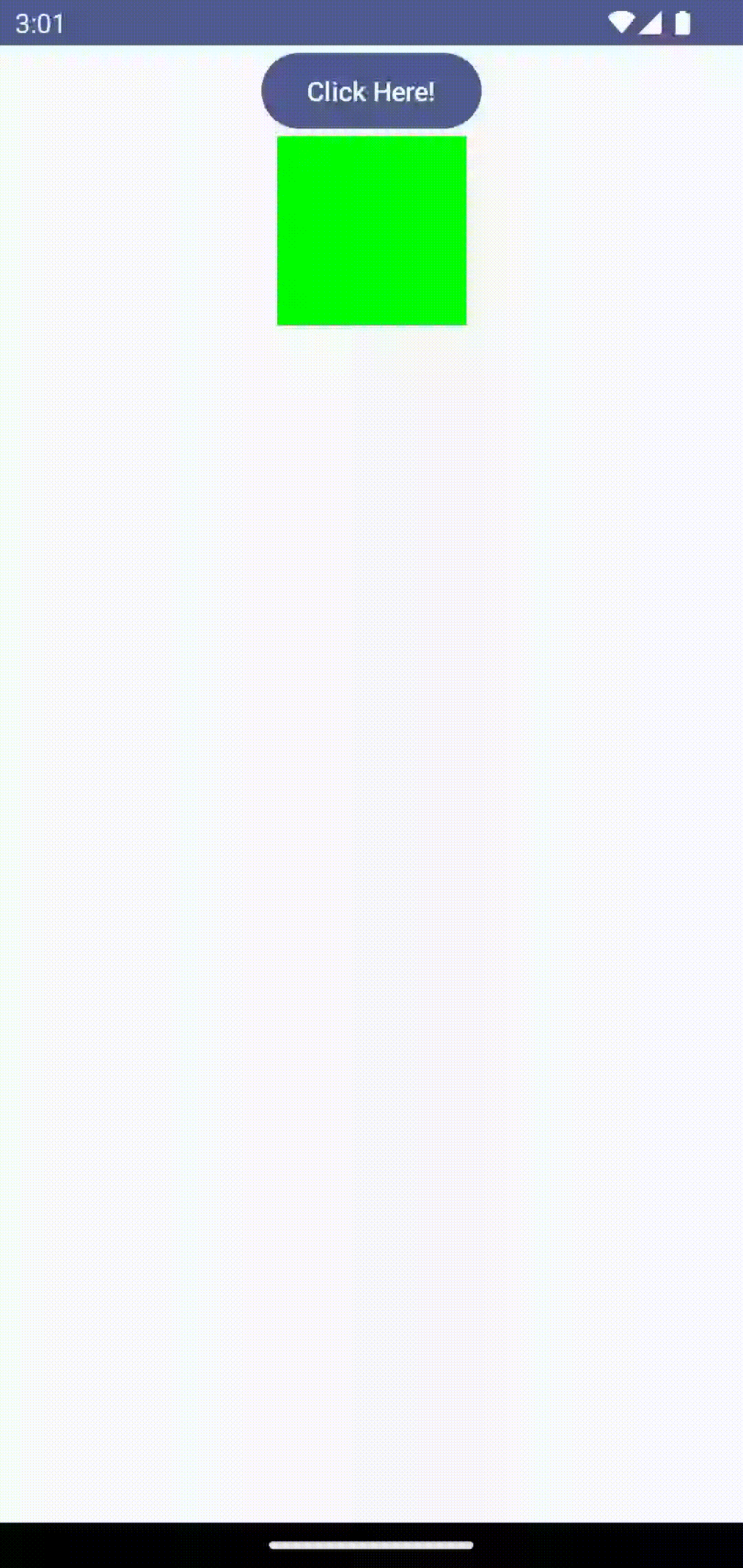
That’s how you animate color in Jetpack Compose.