How to Disable a Button in Android Jetpack Compose
Disabling a button can prevent the user from interacting with a button that is not currently available or relevant. It also helps to prevent errors or unintended actions from occurring. In this blog post, let’s check how to disable a button in Jetpack Compose.
Disabling Button composable is pretty easy. The Button has enabled parameter and all you need is to set it as false.
See the code snippet given below.
Button(enabled = false, onClick = {}) {
Text("Example")
}
The Button turns to a gray color and you will get the following output.
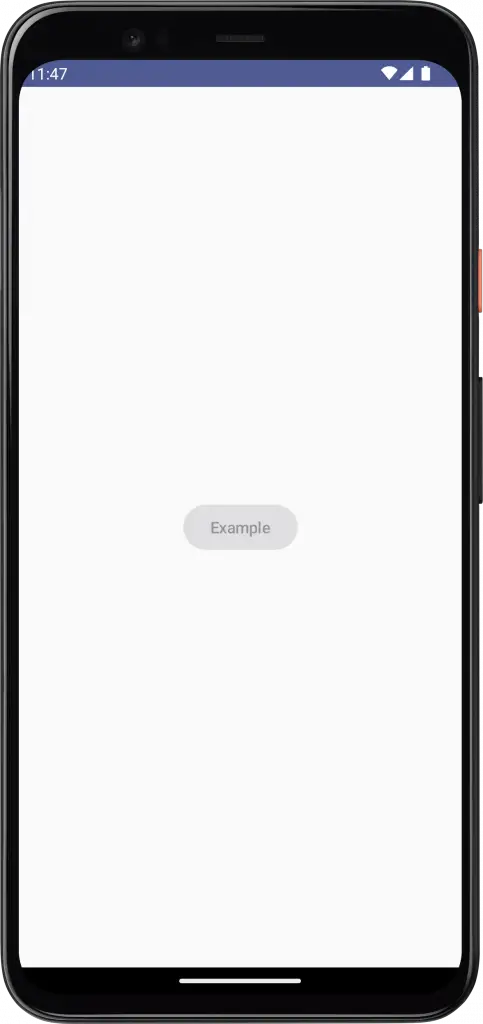
We used a material 3 button in the example.
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ButtonExample()
}
}
}
}
}
@Composable
fun ButtonExample() {
Column(modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center) {
Button(enabled = false, onClick = {}) {
Text("Example")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ButtonExample()
}
}
That’s how you disable a button in Jetpack Compose.