How to Create Gradient Button in Android Jetpack Compose
Gradients are a powerful tool in design and can add depth, dimension, and visual interest to your user interface. When it comes to buttons, gradients can be used to add a subtle touch of elegance and make them stand out from the rest of the interface.
In this blog post, let’s learn how to create a gradient button in Jetpack Compose. You have to use Button composable and Box composable to get the desired result. I am using Material 3 themed compose activity in this example.
Create a new Kotlin file named GradientButton.kt and add the following code to it.
package com.example.myapplication
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.PaddingValues
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Button
import androidx.compose.material3.ButtonDefaults
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
@Composable
fun GradientButton(
text: String,
textColor: Color,
gradient: Brush,
onClick: () -> Unit
) {
Button(
colors = ButtonDefaults.buttonColors(
Color.Transparent
),
contentPadding = PaddingValues(),
onClick = { onClick() })
{
Box(
modifier = Modifier
.background(gradient)
.padding(horizontal = 16.dp, vertical = 16.dp),
contentAlignment = Alignment.Center
) {
Text(text = text, color = textColor)
}
}
}
Now, you can use the GradientButton anywhere. See the following code of MainActivity.kt.
package com.example.myapplication
import android.os.Bundle
import android.util.Log
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ButtonExample()
}
}
}
}
}
@Composable
fun ButtonExample() {
Column(modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center) {
GradientButton(text = "Gradient Button",
onClick = { Log.d("button","clicked")},
textColor = Color.Black,
gradient = Brush.verticalGradient(
0.0f to Color.Green,
1.0f to Color.Cyan,
) )
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ButtonExample()
}
}
You will get the following output.
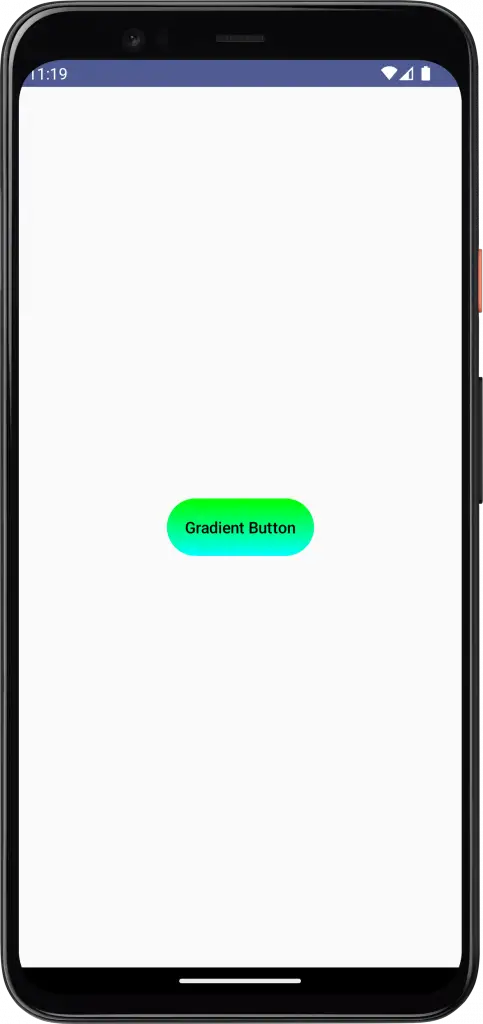
That’s how you add a gradient button in Jetpack Compose.