How to Create Circular Image with Border in Android Jetpack Compose
In today’s mobile apps, circular images have become increasingly popular. These are often used for profile pictures, avatars, or other UI elements. In this tutorial, we’ll explore how to implement circular images with a custom border in Android Jetpack Compose.
We’ll go step-by-step through the code and break down each section for easy understanding.
Why Circular Images?
Circular images add a pleasing aesthetic to your UI. They are especially popular in social media apps, messaging platforms, and even in professional profiles. The rounded shape provides a modern, streamlined look that can make your app more visually engaging.
Create a Circular Image in Jetpack Compose
Creating circular images is straightforward with Jetpack Compose. The key ingredient is the CircleShape
object, which helps to clip an image into a circular shape. Let’s dig into the code.
The Code
Here’s a simple example code snippet for creating a circular image with a red border. It is assumed that you already added an image to Android Studio.
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = "Round Image",
contentScale = ContentScale.Crop,
modifier = Modifier
.size(250.dp)
.padding(20.dp)
.clip(CircleShape)
.border(3.dp, Color.Red, CircleShape)
)
}
In this code, we use the Image
composable function, passing several parameters to customize its appearance.
painter = painterResource(R.drawable.pet)
: This specifies the image resource.contentDescription = "Round Image"
: This provides an accessibility description.contentScale = ContentScale.Crop
: This determines how the image fits within its bounds.modifier = Modifier...
: This allows us to add further customizations like size, padding, and border.
Complete Example
Here’s a complete example, including how you might include this ImageExample
function in your app’s main activity:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ImageExample()
}
}
}
}
}
}
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = "Round Image",
contentScale = ContentScale.Crop,
modifier = Modifier
.size(250.dp)
.padding(20.dp)
.clip(CircleShape)
.border(3.dp, Color.Red, CircleShape)
)
}
Output
With this setup, you should get a circular image with a red border as your output. This will add a pleasing, visually distinct element to your app’s UI.
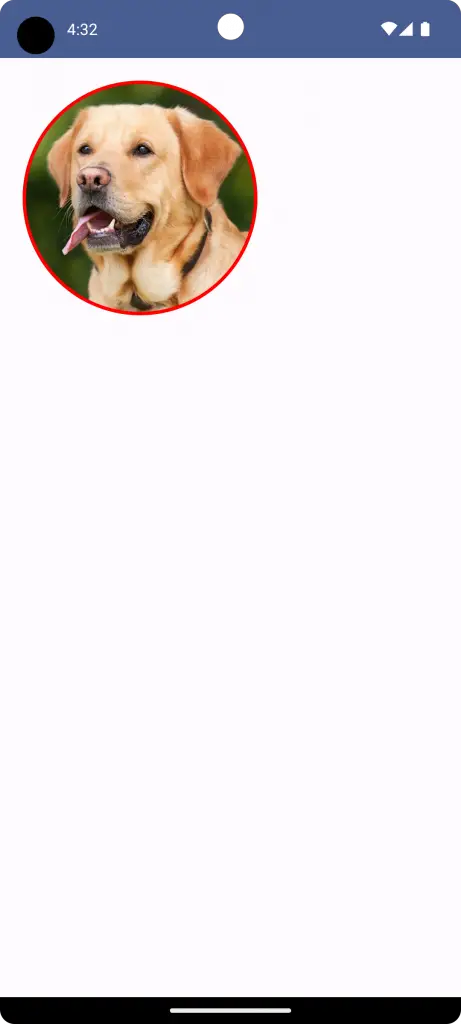
Circular images with custom borders can significantly enhance the look and feel of your Android app. With Jetpack Compose, it’s a breeze to implement this feature. The code is clean, easy to understand, and highly customizable for your specific needs.
One Comment