How to Create Horizontal Gradient in Android Jetpack Compose
In the world of UI design, gradients are a powerful tool to add visual interest and sophistication to your applications. Particularly, horizontal gradients can provide a unique visual transition that draws users in.
In this blog post, we delve into the creation and utilization of horizontal gradients in Android development with Jetpack Compose.
How to Create Horizontal Gradient
A horizontal gradient is a visual transition of colors along the horizontal axis. In Jetpack Compose, we create these gradients using the Brush.horizontalGradient function.
Let’s start with a basic horizontal gradient that transitions from red to blue:
val gradient = Brush.horizontalGradient(
colors = listOf(Color.Red, Color.Blue)
)
In the above example, the gradient starts with red on the left and transitions to blue on the right.
For a gradient that blends more than two colors, just add them to the colors list:
val gradient = Brush.horizontalGradient(
colors = listOf(Color.Red, Color.Yellow, Color.Blue)
)
This gradient transitions from red to yellow and then to blue.
To control the position of each color along the gradient, Jetpack Compose allows you to use color-stop pairs:
val gradient = Brush.horizontalGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.5f to Color.Yellow,
1.0f to Color.Blue
)
)
In this gradient, the color starts with Red on the left (0.0f), transitions to Yellow in the middle (0.5f), and finally to Blue on the right (1.0f).
How to Implement Horizontal Gradient
@Composable
fun GradientExample() {
val gradient = Brush.horizontalGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.5f to Color.Yellow,
1.0f to Color.Blue
)
)
Column() {
Box(modifier = Modifier
.fillMaxWidth().size(150.dp)
.background(gradient))
}
}
Once your gradient is ready, you can use it in any composable that accepts a Brush parameter. For instance, to apply it as the background of a Box.
In this snippet, we use the Modifier.background function to set the Box’s background with our gradient.
Following is the output.
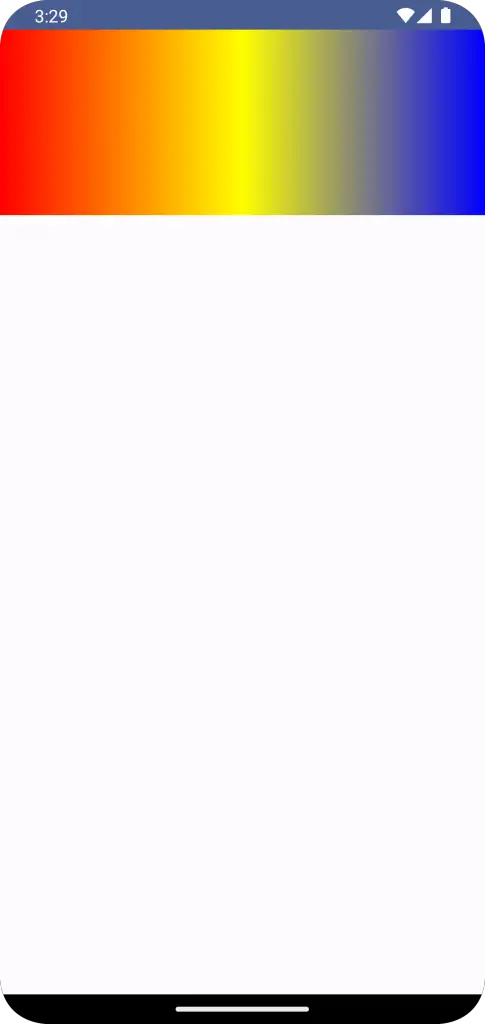
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.size
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
GradientExample()
}
}
}
}
}
@Composable
fun GradientExample() {
val gradient = Brush.horizontalGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.5f to Color.Yellow,
1.0f to Color.Blue
)
)
Column() {
Box(modifier = Modifier
.fillMaxWidth().size(150.dp)
.background(gradient))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
GradientExample()
}
}
Creating horizontal gradients in Jetpack Compose is both straightforward and fun. They are an excellent tool to bring dynamic color transitions to your app, adding to its aesthetic appeal.