How to Create Vertical Gradient in Android Jetpack Compose
As we strive to build visually appealing applications, gradients have emerged as an invaluable tool in UI design. Specifically, vertical gradients provide a captivating visual transition and can enhance the aesthetics of an app.
This blog post aims to guide you through the process of creating and implementing vertical gradients using Jetpack Compose in Android development.
How to Create Vertical Gradient
Vertical gradients transition colors along the vertical axis. In Jetpack Compose, you can create these gradients using the Brush.verticalGradient function.
Let’s start by creating a simple vertical gradient that transitions from red at the top to blue at the bottom.
val gradient = Brush.verticalGradient(
colors = listOf(Color.Red, Color.Blue)
)
In this example, the gradient starts with red at the top and transitions to blue at the bottom.
If you want to create a gradient with more than two colors, simply add more colors to the list:
val gradient = Brush.verticalGradient(
colors = listOf(Color.Red, Color.Yellow, Color.Blue)
)
This gradient transitions from red at the top to yellow in the middle and finally to blue at the bottom.
For more precise control over your gradient, you can specify the position of each color along the gradient using color-stop pairs:
val gradient = Brush.verticalGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.5f to Color.Yellow,
1.0f to Color.Blue
)
)
In this gradient, the color starts with Red at the top (0.0f), transitions to Yellow at the midpoint (0.5f), and finally to Blue at the bottom (1.0f).
How to Implement Vertical Gradient
Once your gradient is defined, it can be applied to any composable that takes a Brush parameter. For example, to set it as the background of a Box.
@Composable
fun GradientExample() {
val gradient = Brush.verticalGradient(
colors = listOf(Color.Red, Color.Yellow, Color.Blue)
)
Column() {
Box(modifier = Modifier
.fillMaxSize()
.background(gradient))
}
}
In this example, we apply the Modifier.background function to set our gradient as the Box’s background. The Modifier.fillMaxSize() function ensures that the Box fills all available space. You will get the following output.
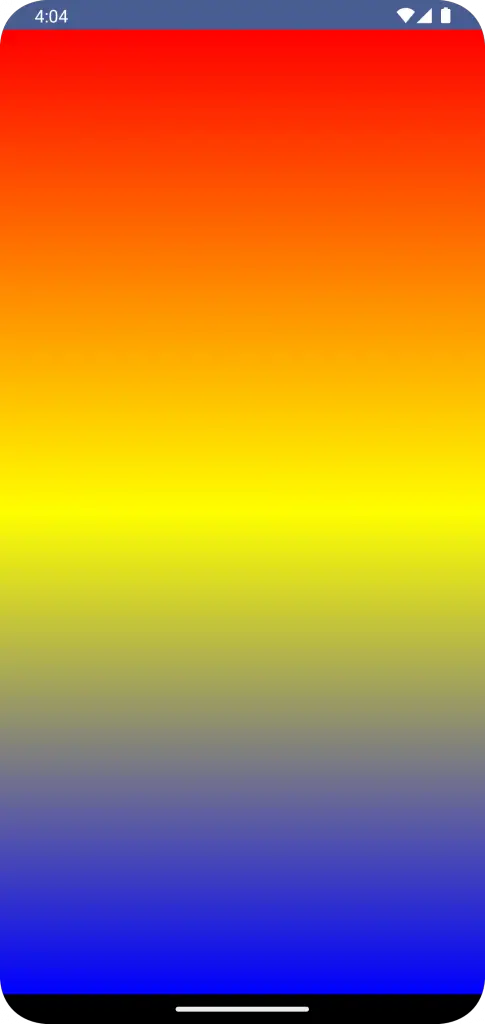
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
GradientExample()
}
}
}
}
}
@Composable
fun GradientExample() {
val gradient = Brush.verticalGradient(
colors = listOf(Color.Red, Color.Yellow, Color.Blue)
)
Column() {
Box(modifier = Modifier
.fillMaxSize()
.background(gradient))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
GradientExample()
}
}
Creating vertical gradients in Jetpack Compose is an intuitive and fun process. They offer a versatile tool for enhancing the visual appeal of your app, bringing dynamic color transitions that can boost your UI’s aesthetics.