How to Add Outlined Button in Android Jetpack Compose
In this tutorial, we will show you how to add an outlined button in Android Jetpack Compose, which is part of the Material 3 design system. Outlined buttons are particularly useful for secondary actions and less important tasks.
The OutlinedButton composable helps to create an outlined button in Jetpack Compose. It has many useful parameters for customization.
See the following code snippet to implement a simple OutlinedButton.
OutlinedButton(onClick = { /*TODO*/ },
border = BorderStroke(width = 1.dp, color = Color.Red)
) {
Text("Outlined Button")
}
You will get an OutlinedButton with a red color border as output.
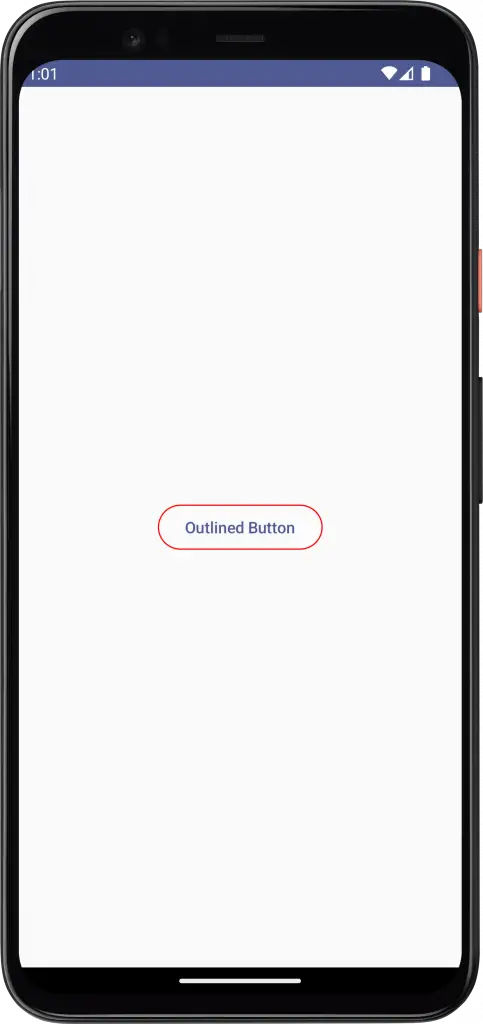
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ButtonExample()
}
}
}
}
}
@Composable
fun ButtonExample() {
Column(modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center) {
OutlinedButton(onClick = { /*TODO*/ },
border = BorderStroke(width = 1.dp, color = Color.Red)
) {
Text("Outlined Button")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ButtonExample()
}
}
That’s how you add an outlined button in Jetpack Compose.
If you need a button with more emphasis, you can also add material 3 ElevatedButton in Jetpack Compose.