How to Change OutlinedTextField Border Color in Android Jetpack Compose
The OutlinedTextField is a widely used composable in Jetpack Compose that creates a Material Design outlined text field for user input. In this blog post, we will explore how to change the border color of an OutlinedTextField in Jetpack Compose.
OutlinedTextField Overview
The OutlinedTextField composable in Jetpack Compose is primarily used to gather user input while adhering to Material Design guidelines. Here’s a basic example of an OutlinedTextField:
val textState = remember { mutableStateOf("") }
OutlinedTextField(
value = textState.value,
onValueChange = { textState.value = it },
label = { Text("Enter your text") },
)
How to Change Border Color
Jetpack Compose’s OutlinedTextField composable provides a colors parameter to change the look of the text field. The focusedBorderColor and unfocusedBorderColor parameters specifically control the border color of the text field when it’s focused (active) and unfocused (inactive), respectively.
Here is how you can change these colors:
fun OutlinedTextFieldExample() {
Column{
val textState = remember { mutableStateOf("") }
OutlinedTextField(
value = textState.value,
onValueChange = { textState.value = it },
label = { Text("Enter your text") },
colors = TextFieldDefaults.outlinedTextFieldColors(
focusedBorderColor = Color.Red,
unfocusedBorderColor = Color.Blue)
)
}
}
In the above example, we’ve set focusedBorderColor to Color.Green and unfocusedBorderColor to Color.Yellow. This means our OutlinedTextField will have a green border when active and a yellow border when inactive.
For more information on TextFieldDefaults.outlinedTextFieldColors, click here. Following is the output.
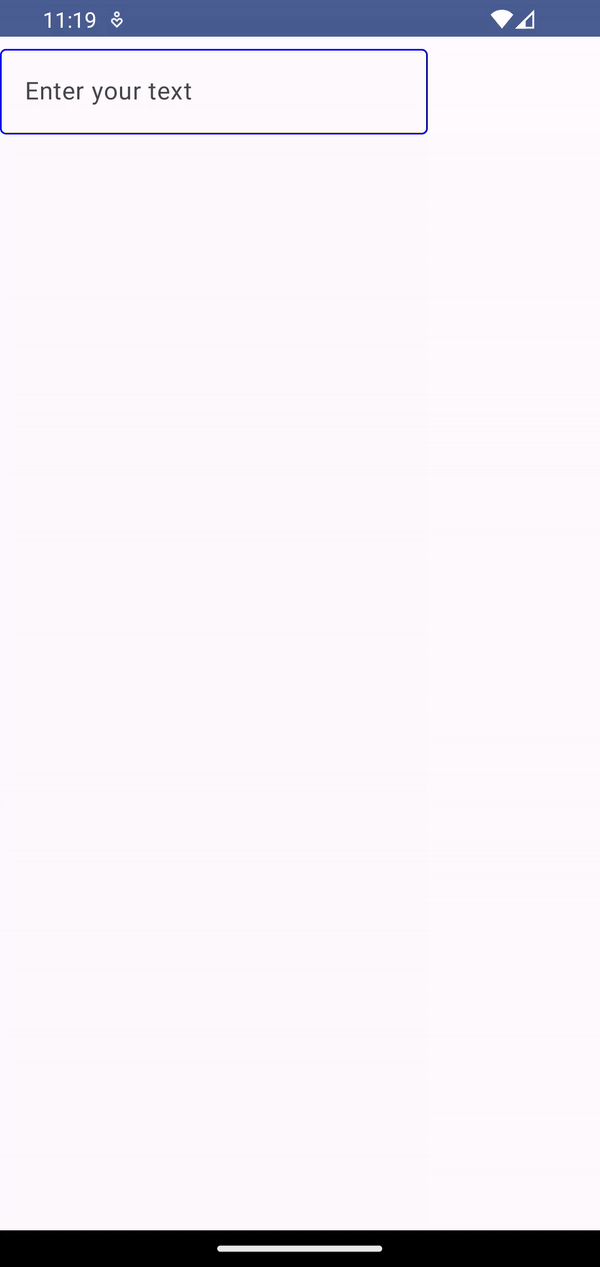
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.OutlinedTextField
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextFieldDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
OutlinedTextFieldExample()
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextFieldExample() {
Column{
val textState = remember { mutableStateOf("") }
OutlinedTextField(
value = textState.value,
onValueChange = { textState.value = it },
label = { Text("Enter your text") },
colors = TextFieldDefaults.outlinedTextFieldColors(
focusedBorderColor = Color.Red,
unfocusedBorderColor = Color.Blue)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
OutlinedTextFieldExample()
}
}
Jetpack Compose allows for a lot of customization of its components. You can easily change the border color of an OutlinedTextField by using the TextFieldDefaults.outlinedTextFieldColors function and the focusedBorderColor and unfocusedBorderColor parameters.
One Comment