Jetpack Compose Switch Colors: Enhance Your App’s UI
Color customization plays a crucial role in creating a visually appealing and brand-consistent UI in Android app development. Jetpack Compose, with its modern UI toolkit, offers extensive flexibility in this area.
In this blog post, we’re focusing on customizing the colors of a Switch
component in Jetpack Compose to align with your app’s theme and style.
Switch in Jetpack Compose
A Switch
in Jetpack Compose is used for toggling between two states, typically ON and OFF. Customizing its colors can significantly enhance user interaction and integrate the component seamlessly into your app’s design.
Customize Switch Colors
By default, a Switch
in Jetpack Compose uses the colors defined in the app’s theme. However, customizing these colors is a common requirement.
Jetpack Compose provides SwitchDefaults.colors
to customize the switch’s thumb and track colors for both checked and unchecked states.
Example:
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
colors = SwitchDefaults.colors(
checkedThumbColor = Color.Green,
uncheckedThumbColor = Color.Gray,
checkedTrackColor = Color.Green.copy(alpha = 0.1f),
uncheckedTrackColor = Color.Gray.copy(alpha = 0.1f)
)
)
In this example, the switch thumb turns green when ON and gray when OFF. Similarly, the track color (the background of the thumb) also changes accordingly, with a reduced alpha for a lighter shade.
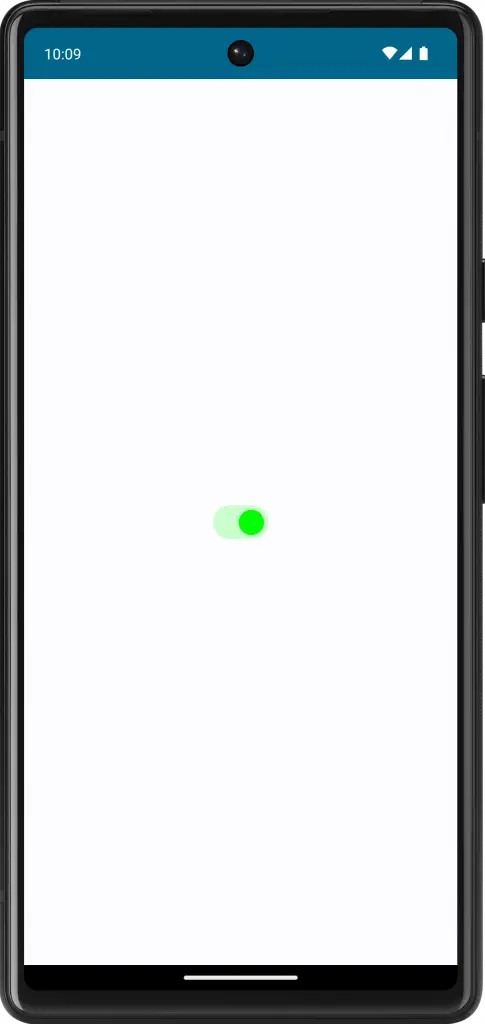
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Switch
import androidx.compose.material3.SwitchDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
MySwitch()
}
}
}
}
}
@Composable
fun MySwitch() {
var isChecked by remember { mutableStateOf(true) }
Switch(
checked = isChecked,
onCheckedChange = { isChecked = it },
colors = SwitchDefaults.colors(
checkedThumbColor = Color.Green,
uncheckedThumbColor = Color.Gray,
checkedTrackColor = Color.Green.copy(alpha = 0.2f),
uncheckedTrackColor = Color.Gray.copy(alpha = 0.2f)
)
)
}
Customizing switch colors in Jetpack Compose is a powerful way to enhance your app’s UI. It allows for greater consistency with your app’s theme and improves the overall user experience.
With simple steps and thoughtful color choices, your switches can become an integral part of your app’s design.