How to Add LazyColumn with Divider in Android Jetpack Compose
In this tutorial, we’ll learn how to add dividers to a LazyColumn in Jetpack Compose, Google’s modern toolkit for building native Android UIs. The LazyColumn widget is particularly useful for displaying lists of items in a memory-efficient manner.
LazyColumn is a composable function that lazily loads and displays a vertical list of items. It only renders what’s currently visible on the screen, which makes it a more efficient choice for large lists compared to the traditional Column.
On the other hand, Divider is a thin horizontal line that’s commonly used to separate items in a list. It provides a clear visual break between individual items, improving readability and enhancing the user experience.
Here’s a simple example of how to add dividers to a LazyColumn:
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material.Divider
import androidx.compose.runtime.Composable
import androidx.compose.ui.graphics.Color
@Composable
fun MyScreen() {
val listItems = (1..20).toList()
LazyColumn {
items(listItems) { item ->
Text(text = "Item $item")
Divider(color = Color.Black)
}
}
}
In this code snippet, we’ve created a LazyColumn with a list of 20 items. For each item, we display a Text widget followed by a Divider. The Divider is drawn with a black color.
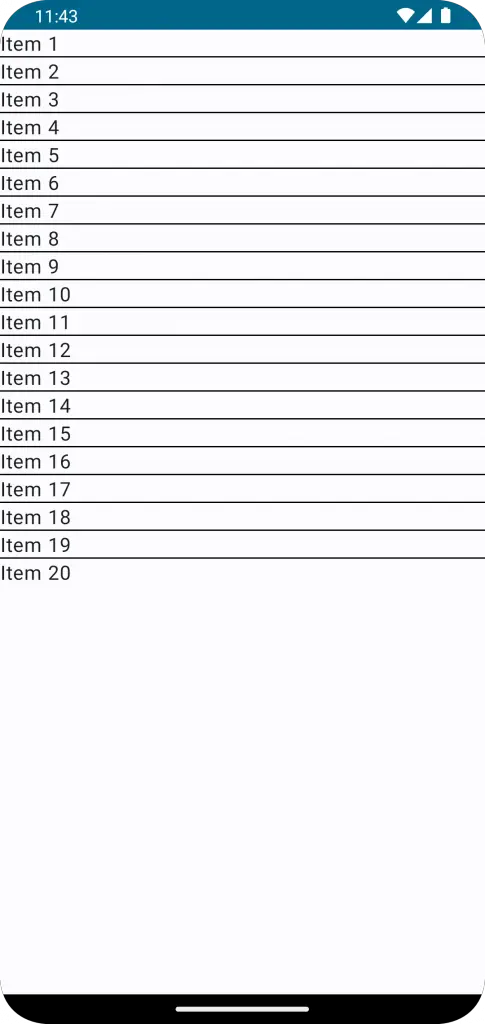
By incorporating dividers into your LazyColumn, you can make your list-based UIs more organized and visually appealing.