How to make TextField Number only in Android Jetpack Compose
TextField is the field where the user types in. Sometimes developers want to show a specific type of keyboard to enter data. This Jetpack Compose tutorial helps you to make the TextField number only.
KeyboardOptions class helps you to choose different keyboard types for TextField.
@Composable
fun Example() {
var value by remember { mutableStateOf("") }
TextField(
value = value,
onValueChange = { value = it },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Number),
label = { Text("Enter text") },
maxLines = 2,
modifier = Modifier.padding(20.dp)
)
}
KeyboardType.Number will show the keyboard as given in the screenshot.
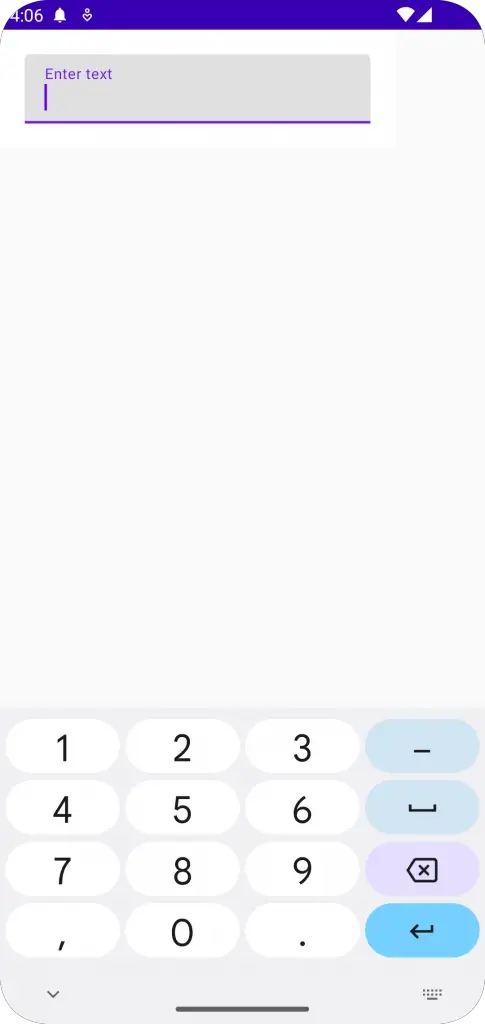
If you want a keyboard type for a number password then you have to use KeyboardType.NumberPassword.
@Composable
fun Example() {
var value by remember { mutableStateOf("") }
TextField(
value = value,
onValueChange = { value = it },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.NumberPassword),
label = { Text("Enter text") },
maxLines = 2,
modifier = Modifier.padding(20.dp)
)
}
Then you will get the following keyboard without characters such as , . etc.
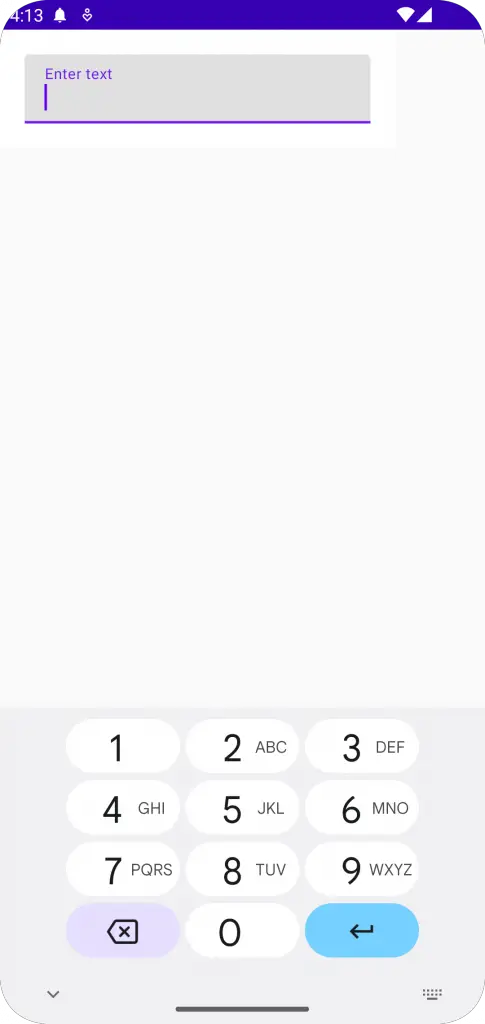
In many cases, you may need a phone keyboard type to enter a mobile phone number. Use KeyboardType.Phone to get the desired result.
@Composable
fun Example() {
var value by remember { mutableStateOf("") }
TextField(
value = value,
onValueChange = { value = it },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Phone),
label = { Text("Enter text") },
maxLines = 2,
modifier = Modifier.padding(20.dp)
)
}
Following is the output.
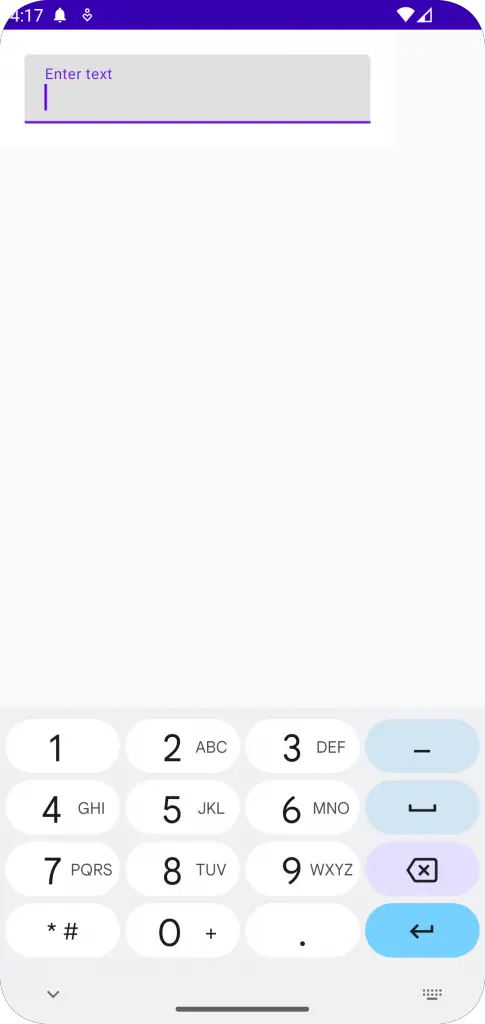
Following is the complete code for your reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.input.KeyboardType
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Sample Text") },
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Phone),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
That’s how you add number keyboard type and phone keyboard type in Jetpack Compose.
One Comment