How to Create Capsule Shape in SwiftUI
In SwiftUI, a Capsule is a shape that can be considered a rounded rectangle with fully curved shorter sides, resembling a pill shape. It’s useful for creating button backgrounds, on/off toggle graphics, and more.
This blog post will give you an in-depth look at the Capsule shape in SwiftUI, and how to use it effectively in your UI designs.
Capsule Shape
The Capsule shape is a part of SwiftUI’s library of basic shapes, alongside Rectangles, Circles, and Ellipses. It’s a versatile shape that can add a modern and user-friendly touch to your app’s interface.
Basic Capsule
Creating a Capsule in SwiftUI is straightforward:
import SwiftUI
struct ContentView: View {
var body: some View {
Capsule()
.frame(width: 200, height: 100)
.foregroundColor(.green)
}
}
In this example, we initialize a Capsule and set its frame to be 200 points wide and 100 points tall. We also set the foreground color to green. This will create a green Capsule that’s twice as wide as it is tall.
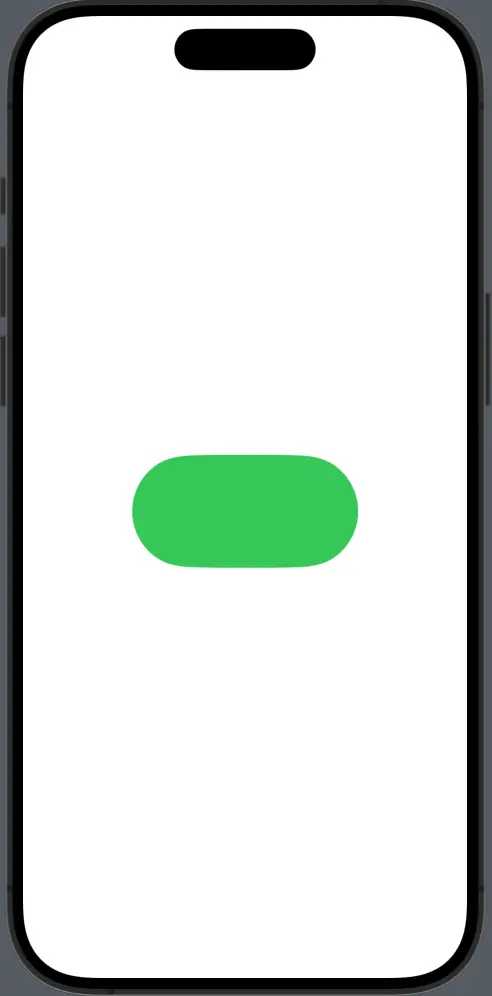
Style Capsule Shape
Add Borders
To add a border to a Capsule, you can use the .overlay()
modifier with another Capsule that’s only a stroke.
Capsule()
.frame(width: 200, height: 100)
.foregroundColor(.blue)
.overlay(
Capsule().stroke(Color.red, lineWidth: 4)
)
The blue Capsule now has a red border that’s 4 points thick.
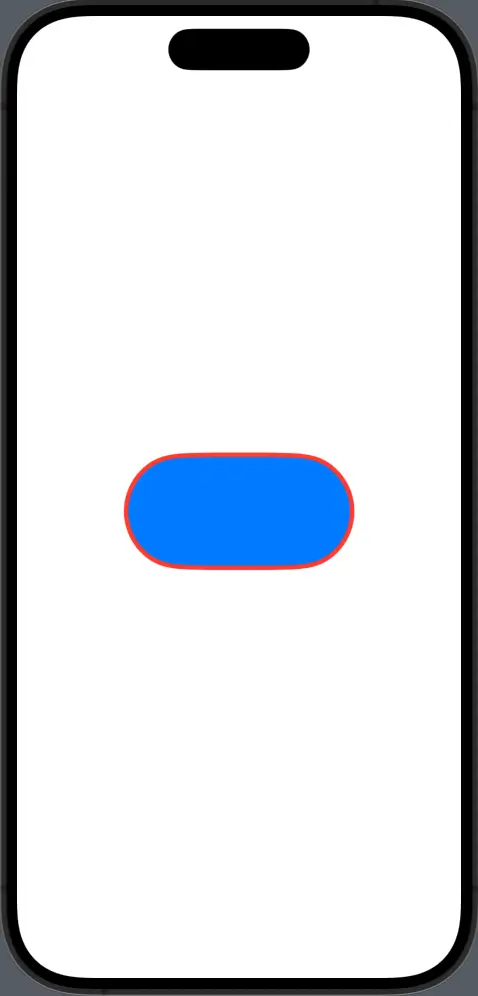
Capsule as Buttons
One common use for the Capsule shape is as a background for buttons and toggle switches.
Button(action: {
// Perform an action
}) {
Text("Get Started")
.font(.headline)
.foregroundColor(.white)
.padding()
.background(Capsule().fill(Color.blue))
}
Here, a button with the text “Get Started” is embedded in a blue Capsule, making for a stylish and tappable button.
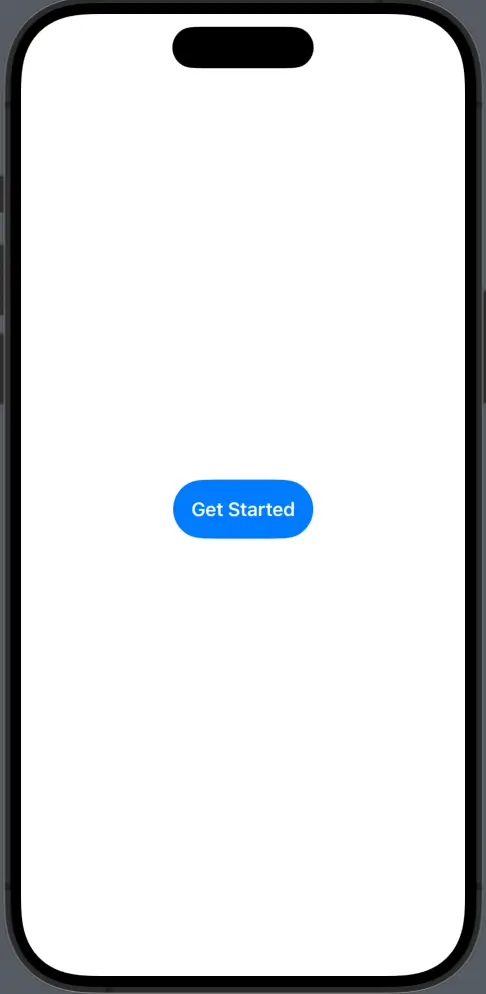
The Capsule shape is an essential component in the SwiftUI toolkit. Its pleasing shape is perfect for creating elements that users will want to interact with. By adding colors and borders you can create visually engaging UI components that are both beautiful and functional.